NumPy: Create an array of 10 zeros, 10 ones, 10 fives
NumPy: Basic Exercise-13 with Solution
Create Array of Zeros, Ones, Fives
Write a NumPy program to create an array of 10 zeros, 10 ones, and 10 fives.
This NumPy program creates three separate arrays: one consisting of 10 zeros, another of 10 ones, and the last of 10 fives. It utilizes NumPy's array creation functions to generate these arrays efficiently. This program demonstrates how to initialize arrays with specific values in a concise manner.
Sample Solution :
Python Code :
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating an array of 10 zeros using np.zeros()
array = np.zeros(10)
# Printing a message indicating an array of 10 zeros
print("An array of 10 zeros:")
# Printing the array of 10 zeros
print(array)
# Creating an array of 10 ones using np.ones()
array = np.ones(10)
# Printing a message indicating an array of 10 ones
print("An array of 10 ones:")
# Printing the array of 10 ones
print(array)
# Creating an array of 10 fives by multiplying an array of 10 ones by 5
array = np.ones(10) * 5
# Printing a message indicating an array of 10 fives
print("An array of 10 fives:")
# Printing the array of 10 fives
print(array)
Output:
An array of 10 zeros: [ 0. 0. 0. 0. 0. 0. 0. 0. 0. 0.] An array of 10 ones: [ 1. 1. 1. 1. 1. 1. 1. 1. 1. 1.] An array of 10 fives: [ 5. 5. 5. 5. 5. 5. 5. 5. 5. 5.]
Explanation:
In the said code -
The np.zeros(10) function creates a NumPy array with 10 elements, all initialized to the value 0. The default data type is float64.
The np.ones(10) function creates a new NumPy array with 10 elements, all initialized to the value 1. The default data type is float64.
The np.ones(10)*5 function creates a new NumPy array with 10 elements, all initialized to the value 1, and then multiplies each element by 5. The resulting array will have all elements equal to 5. The default data type is float64.
Visual Presentation:
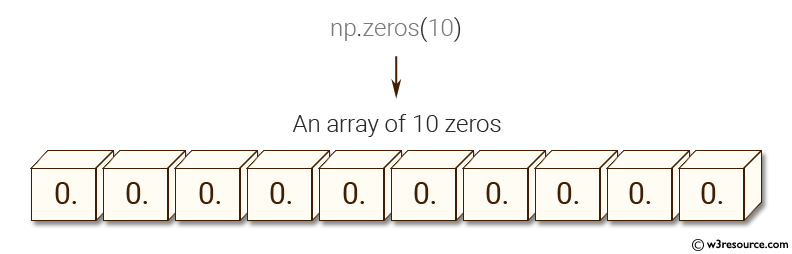
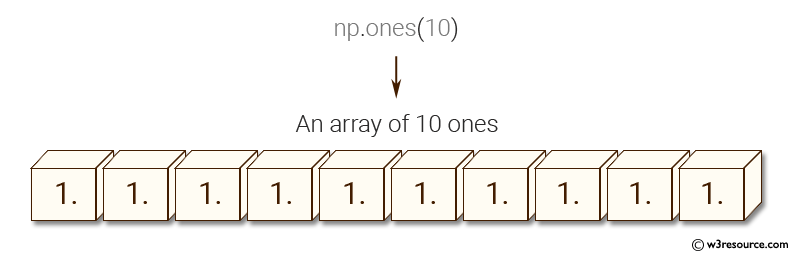
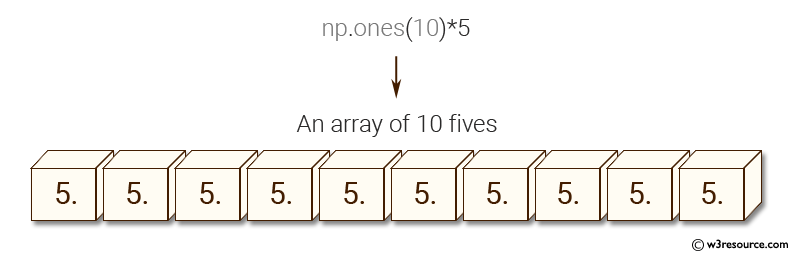
Python-Numpy Code Editor:
Previous: NumPy program to create an array with the values 1, 7, 13, 105 and determine the size of the memory occupied by the array.
Next: NumPy program to create an array of the integers from 30 to70.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/numpy/basic/numpy-basic-exercise-13.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics