NumPy: Create an array of the integers from 30 to 70
Create Array of Integers 30 to 70
Write a NumPy program to create an array of integers from 30 to 70.
This NumPy program generates an array of integers ranging from 30 to 70. By using NumPy's array creation functions, it efficiently constructs the array with the specified sequence of integers. This program highlights how to create arrays with defined ranges in a straightforward manner.
Sample Solution :
Python Code :
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating an array of integers from 30 to 70 using np.arange()
array = np.arange(30, 71)
# Printing a message indicating an array of integers from 30 to 70
print("Array of the integers from 30 to 70")
# Printing the array of integers from 30 to 70
print(array)
Output:
Array of the integers from 30 to70 [30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70]
Explanation:
In the above code -
array=np.arange(30,71): The np.arange() function creates a NumPy array containing values within a specified interval. In this case, it generates an array starting at 30 and ending before 71, with a default step size of 1. This results in an array of integers from 30 to 70. Since the step size is not explicitly specified, it defaults to 1.
The resulting 'array' will contain the integers from 30 to 70, inclusive: [30 31 32 33 ... 68 69 70].
Visual Presentation:
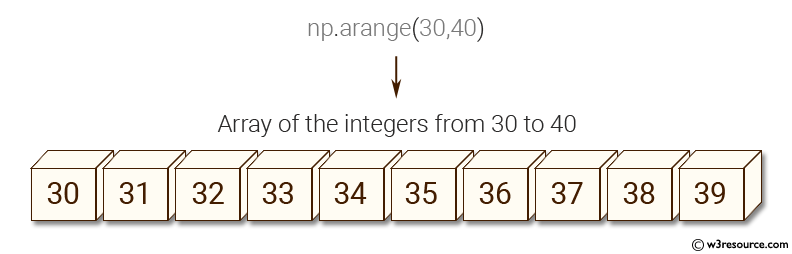
For more Practice: Solve these Related Problems:
- Generate a 2D array where each row is a sequence of integers from 30 to 70.
- Create an array of integers from 30 to 70 and then output the reversed sequence.
- Form an array from 30 to 70 using np.arange and verify the inclusion of both endpoints.
- Generate a sequential array from 30 to 70 with a custom step and adjust it to include 70.
Go to:
PREV : Create Array of Zeros, Ones, Fives
NEXT : Create Array of Even Integers 30 to 70
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.