NumPy: Find the number of rows and columns of a given matrix
Rows & Columns in Matrix
Write a NumPy program to find the number of rows and columns in a given matrix.
This problem entails writing a NumPy program to determine the number of rows and columns in a given matrix. The task involves utilizing NumPy's array attributes to extract the dimensions of the matrix, providing insights into its structure. By accessing the shape attribute of the array, the program efficiently identifies the number of rows and columns in the matrix.
Sample Solution :
Python Code :
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a NumPy array 'm' containing integers from 10 to 21 and reshaping it into a 3x4 matrix using np.arange() and .reshape()
m = np.arange(10, 22).reshape((3, 4))
# Printing a message indicating the original matrix 'm'
print("Original matrix:")
print(m)
# Printing a message indicating the number of rows and columns of the matrix 'm' using the .shape attribute
print("Number of rows and columns of the said matrix:")
print(m.shape)
Output:
Original matrix: [[10 11 12 13] [14 15 16 17] [18 19 20 21]] Number of rows and columns of the said matrix: (3, 4)
Explanation:
In the above code the np.arange() function generates an array of evenly spaced values within a specified interval. In this case, it generates an array starting at 10 and ending before 22, with a default step size of 1, resulting in an array of integers from 10 to 21. Then, the reshape() method is used to change the shape of the generated array into a 3x4 matrix (3 rows and 4 columns).
Then print(m) prints the reshaped array 'm' to the console. The output will be a 3x4 matrix containing integers from 10 to 21.:
Finally print(m.shape) prints the shape of the array 'm' to the console.
Visual Presentation:
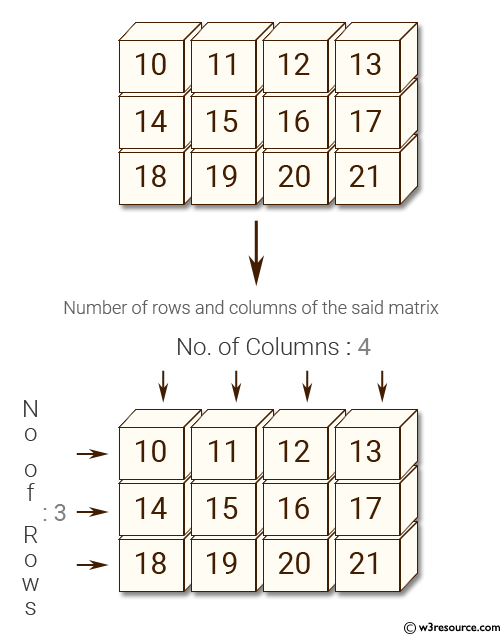
For more Practice: Solve these Related Problems:
- Determine the shape of a given matrix and print the number of rows.
- Extract and print the number of columns from a randomly generated 2D array.
- Write a function that returns both the row and column count for an input matrix.
- Given a matrix, verify if it is square by comparing its row and column counts.
Go to:
Previous: NumPy program to create a 3x4 matrix filled with values from 10 to 21.
Next: NumPy program to create a 3x3 identity matrix, i.e. diagonal elements are 1, the rest are 0.
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.