NumPy: Create a 3x3 identity matrix, i.e. diagonal elements are 1,the rest are 0
Create 3x3 Identity Matrix
Write a NumPy program to create a 3x3 identity matrix, i.e. the diagonal elements are 1, the rest are 0.
This problem involves writing a NumPy program to generate a 3x3 identity matrix, where all diagonal elements are 1 and the rest are 0. The task requires leveraging NumPy's identity function, which efficiently constructs such matrices of any desired size with the specified diagonal values. The resulting identity matrix aids in various mathematical operations, such as linear transformations and solving systems of equations.
Sample Solution :
Python Code :
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a 3x3 identity matrix using np.eye()
x = np.eye(3)
# Printing the created 3x3 identity matrix 'x'
print(x)
Output:
[[ 1. 0. 0.] [ 0. 1. 0.] [ 0. 0. 1.]]
Explanation:
An identity matrix is a square matrix with ones on its main diagonal and zeros elsewhere.
In the above code the line ‘x = np.eye(3)’ uses the np.eye() function to create a 2-dimensional square identity matrix 'x' of size 3x3.
Finally print(x) prints the generated identity matrix to the console.
Visual Presentation:
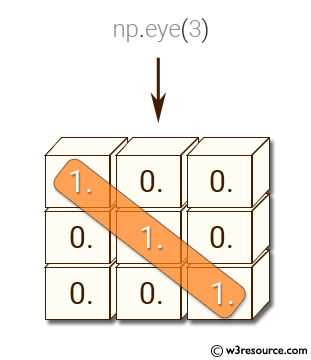
For more Practice: Solve these Related Problems:
- Generate a 3x3 identity matrix using a built-in NumPy function and validate its diagonal.
- Create a 3x3 matrix filled with zeros and set its diagonal to ones using slicing.
- Construct an identity matrix and compare it with the output of np.eye.
- Form a 3x3 identity matrix and compute its determinant to verify correctness.
Go to:
Previous: NumPy program to find the number of rows and columns of a given matrix.
Next: NumPy program to create a 10x10 matrix, in which the elements on the borders will be equal to 1, and inside 0.
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.