NumPy: Create a 10x10 matrix, in which the elements on the borders will be equal to 1, and inside 0
10x10 Matrix Border 1, Inside 0
Write a NumPy program to create a 10x10 matrix, in which the elements on the borders will be equal to 1, and inside 0.
This problem involves writing a NumPy program to generate a 10x10 matrix where the border elements are set to 1 and the inner elements are set to 0. The task requires utilizing NumPy's array manipulation capabilities to efficiently create the matrix with the specified values. By setting the border elements to 1 and the inner elements to 0, the resulting matrix represents a common pattern used in various computational and mathematical contexts.
Sample Solution :
Python Code :
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a 10x10 matrix filled with ones using np.ones()
x = np.ones((10, 10))
# Setting the inner values of the matrix 'x' (excluding the borders) to 0 using slicing
x[1:-1, 1:-1] = 0
# Printing the modified matrix 'x'
print(x)
Output:
[[ 1. 1. 1. 1. 1. 1. 1. 1. 1. 1.] [ 1. 0. 0. 0. 0. 0. 0. 0. 0. 1.] [ 1. 0. 0. 0. 0. 0. 0. 0. 0. 1.] [ 1. 0. 0. 0. 0. 0. 0. 0. 0. 1.] [ 1. 0. 0. 0. 0. 0. 0. 0. 0. 1.] [ 1. 0. 0. 0. 0. 0. 0. 0. 0. 1.] [ 1. 0. 0. 0. 0. 0. 0. 0. 0. 1.] [ 1. 0. 0. 0. 0. 0. 0. 0. 0. 1.] [ 1. 0. 0. 0. 0. 0. 0. 0. 0. 1.] [ 1. 1. 1. 1. 1. 1. 1. 1. 1. 1.]]
Explanation:
In the above code -
np.ones((10, 10)) creates a 10x10 array 'x' filled with ones.
‘x[1:-1, 1:-1] = 0’ uses array slicing to select the inner region of the array 'x' and set its elements to zeros. The slice 1:-1 means "from the second element to the second-to-last element" in both the rows and columns. As a result, the inner region of the array will be filled with zeros, leaving a border of ones.
Finally print(x) prints the modified array to the console.
Visual Presentation:
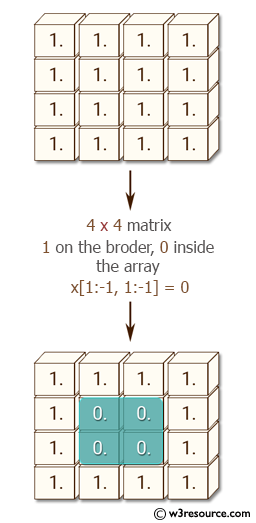
For more Practice: Solve these Related Problems:
- Create a 10x10 matrix with ones on the border and zeros inside, then calculate the sum of the border elements.
- Generate a matrix with a border of ones and fill the inside with sequential numbers.
- Construct a 10x10 hollow matrix with border ones, then invert the inner submatrix.
- Create a matrix with border ones and inner zeros, then swap the top and bottom border rows.
Go to:
Previous: NumPy program to create a 3x3 identity matrix, i.e. diagonal elements are 1,the rest are 0.
Next: NumPy program to create a 5x5 zero matrix with elements on the main diagonal equal to 1, 2, 3, 4, 5.
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.