NumPy: Create a 5x5 zero matrix with elements on the main diagonal equal to 1, 2, 3, 4, 5
NumPy: Basic Exercise-29 with Solution
Diagonal 5x5 Matrix (1-5)
Write a NumPy program to create a 5x5 zero matrix with elements on the main diagonal equal to 1, 2, 3, 4, 5.
This problem involves writing a NumPy program to generate a 5x5 matrix where all elements are initially set to zero, except for the main diagonal, which is filled with the values 1, 2, 3, 4, and 5. The task requires utilizing NumPy's array manipulation capabilities to efficiently construct and modify the matrix, ensuring the specified values are placed correctly on the diagonal. The resulting matrix combines elements of identity matrices with custom diagonal values.
Sample Solution :
Python Code :
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a diagonal matrix with diagonal elements 1, 2, 3, 4, 5 using np.diag()
x = np.diag([1, 2, 3, 4, 5])
# Printing the diagonal matrix 'x'
print(x)
Output:
[[1 0 0 0 0] [0 2 0 0 0] [0 0 3 0 0] [0 0 0 4 0] [0 0 0 0 5]]
Explanation:
The above exercise creates a 5x5 square matrix with the main diagonal elements [1, 2, 3, 4, 5] and zeros elsewhere, and prints the resulting matrix.
In the above code np.diag([1, 2, 3, 4, 5]) creates a 2D square matrix with the specified diagonal elements [1, 2, 3, 4, 5]. The rest of the elements in the matrix are filled with zeros.
Finally print(x) prints the generated matrix to the console.
Visual Presentation:
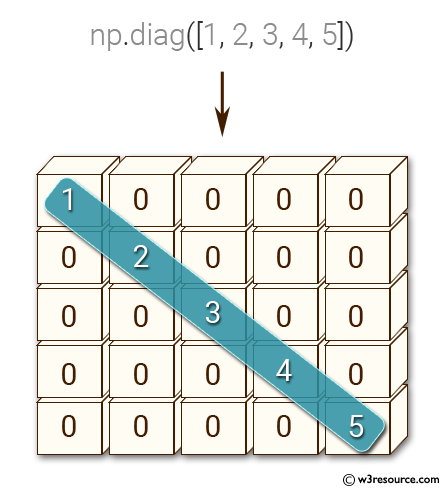
Python-Numpy Code Editor:
Previous: NumPy program to create a 10x10 matrix, in which the elements on the borders will be equal to 1, and inside 0.
Next: NumPy program to create an 4x4 matrix in which 0 and 1 are staggered, with zeros on the main diagonal.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/numpy/basic/numpy-basic-exercise-29.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics