NumPy: Create a 4x4 matrix in which 0 and 1 are staggered, with zeros on the main diagonal
Staggered 4x4 Matrix (0,1)
Write a NumPy program to create a 4x4 matrix in which 0 and 1 are staggered, with zeros on the main diagonal.
This problem involves writing a NumPy program to generate a 4x4 matrix where zeros are placed on the main diagonal, and ones and zeros alternate off the diagonal. The task requires utilizing NumPy's array manipulation capabilities to efficiently construct the matrix with the specified pattern. By staggering the arrangement of zeros and ones, while ensuring zeros are on the main diagonal, the resulting matrix exhibits a distinctive alternating pattern.
Sample Solution :
Python Code :
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a 4x4 matrix filled with zeros using np.zeros()
x = np.zeros((4, 4))
# Setting elements in alternate rows and columns to 1
# Setting elements in even rows (0-based indexing) and odd columns to 1
x[::2, 1::2] = 1
# Setting elements in odd rows and even columns to 1
x[1::2, ::2] = 1
# Printing the modified matrix 'x'
print(x)
Output:
[[ 0. 1. 0. 1.] [ 1. 0. 1. 0.] [ 0. 1. 0. 1.] [ 1. 0. 1. 0.]]
Explanation:
In the above code -
In ‘x = np.zeros((4, 4))’ statement the np.zeros() function is used to create a 4x4 array 'x' filled with zeros.
x[::2, 1::2] = 1: This line uses array slicing to select every other row (starting from the first row) and every other column (starting from the second column) in the array 'x' and sets their elements to ones. The slice ::2 means "every second element" in both the rows and columns, while the slice 1::2 means "every second element, starting from the second element".
x[1::2, ::2] = 1: This line uses array slicing to select every other row (starting from the second row) and every other column (starting from the first column) in the array 'x' and sets their elements to ones. The slice 1::2 means "every second element, starting from the second element" in both the rows and columns.
Finally print(x) prints the modified array to the console.
Visual Presentation:
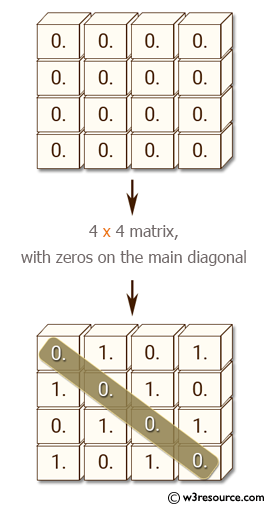
For more Practice: Solve these Related Problems:
- Generate a 4x4 matrix with alternating 0s and 1s starting with 0 on the main diagonal.
- Create a staggered pattern matrix where even rows are filled with zeros and odd rows with ones.
- Construct a 4x4 matrix displaying a checkerboard pattern of 0s and 1s.
- Generate a 4x4 matrix with 0 on the main diagonal and alternating values in off-diagonals.
Go to:
PREV : Diagonal 5x5 Matrix (1-5)
NEXT : Create 3x3x3 Array of Values
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.