NumPy: Test whether none of the elements of a given array is zero
Test None are Zero
Write a NumPy program to test whether none of the elements of a given array are zero.
This problem involves using NumPy to perform an element-wise check on an array. The task is to write a program that determines whether none of the elements in a given NumPy array are zero. This is useful for validating data where the presence of zero values might be problematic or indicate an error.
Sample Solution :
Python Code :
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a NumPy array 'x' containing elements 1, 2, 3, and 4
x = np.array([1, 2, 3, 4])
# Printing the original array 'x'
print("Original array:")
print(x)
# Checking if none of the elements in the array 'x' is zero and printing the result
print("Test if none of the elements of the said array is zero:")
print(np.all(x))
# Reassigning array 'x' to a new array containing elements 0, 1, 2, and 3
x = np.array([0, 1, 2, 3])
# Printing the new array 'x'
print("Original array:")
print(x)
# Checking if none of the elements in the updated array 'x' is zero and printing the result
print("Test if none of the elements of the said array is zero:")
print(np.all(x))
Output:
Original array: [1 2 3 4] Test if none of the elements of the said array is zero: True Original array: [0 1 2 3] Test if none of the elements of the said array is zero: False
Explanation:
In the above exercise -
x = np.array([1, 2, 3, 4]): This line creates a NumPy array 'x' with the elements 1, 2, 3, and 4.
print(np.all(x)): This line uses the np.all() function to test if all elements in the array 'x' are non-zero (i.e., not equal to zero). In this case, all elements are non-zero, so the function returns True.
x = np.array([0, 1, 2, 3]): This line creates a new NumPy array 'x' with the elements 0, 1, 2, and 3.
print(np.all(x)): This line uses the np.all() function again to test if all elements in the new array 'x' are non-zero. In this case, the first element is zero, so the function returns False.
Pictorial Presentation:
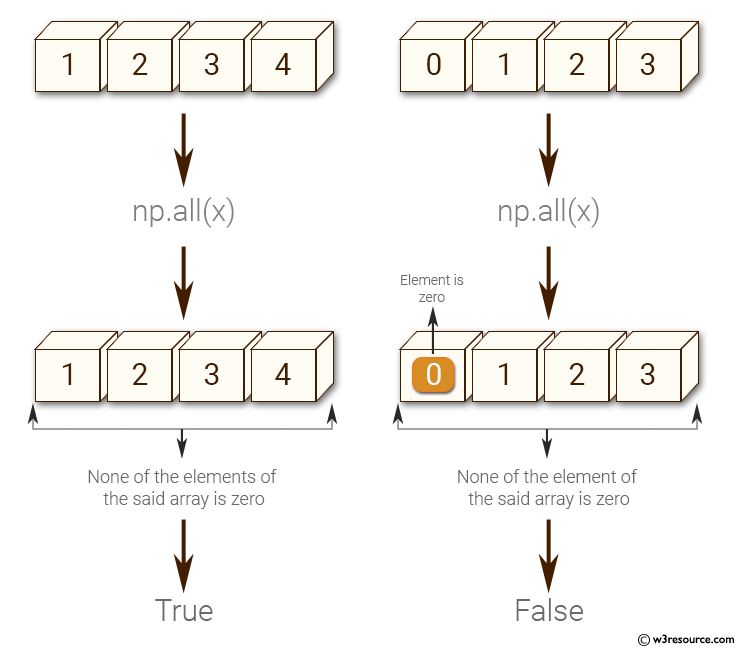
For more Practice: Solve these Related Problems:
- Check if an array contains no zeros using vectorized logical operations.
- Create a boolean mask that verifies the absence of zero values in a multi-dimensional array.
- Test whether the product of all elements in an array is non-zero without using built-in functions.
- Implement a custom function using np.all to ensure that no element equals zero.
Go to:
PREV : Help for NumPy Add Function
NEXT : Test Any Non-Zero
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.