NumPy: Test whether any of the elements of a given array is non-zero
NumPy: Basic Exercise-4 with Solution
Test Any Non-Zero
Write a NumPy program to test if any of the elements of a given array are non-zero.
This problem involves using NumPy to perform a check on the elements of an array. The task is to write a program that determines if any of the elements in a given NumPy array are non-zero. This is useful for situations where the presence of non-zero values is significant, such as checking for valid data entries or detecting activity in datasets.
Sample Solution :
Python Code :
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a NumPy array 'x' containing elements 1, 0, 0, and 0
x = np.array([1, 0, 0, 0])
# Printing the original array 'x'
print("Original array:")
print(x)
# Checking if any element in the array 'x' is non-zero and printing the result
print("Test whether any of the elements of a given array is non-zero:")
print(np.any(x))
# Reassigning array 'x' to a new array containing all elements as zero
x = np.array([0, 0, 0, 0])
# Printing the new array 'x'
print("Original array:")
print(x)
# Checking if any element in the updated array 'x' is non-zero and printing the result
print("Test whether any of the elements of a given array is non-zero:")
print(np.any(x))
Output:
Original array: [1 0 0 0] Test whether any of the elements of a given array is non-zero: True Original array: [0 0 0 0] Test whether any of the elements of a given array is non-zero: False
Explanation:
In the above code –
x = np.array([1, 0, 0, 0]): This line creates a NumPy array 'x' with the elements 1, 0, 0, and 0.
print(np.any(x)): This line uses the np.any() function to test if any elements in the array 'x' are non-zero (i.e., not equal to zero). In this case, the first element is non-zero, so the function returns True.
x = np.array([0, 0, 0, 0]): This line creates a new NumPy array 'x' with all elements equal to 0.
print(np.any(x)): This line uses the np.any() function again to test if any elements in the new array 'x' are non-zero. In this case, all elements are zero, so the function returns False.
Visual Presentation:
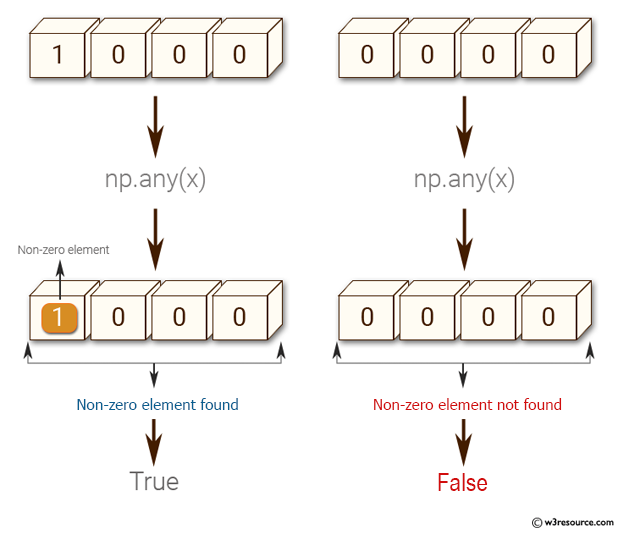
Python-Numpy Code Editor:
Previous: Test whether none of the elements of a given array is zero.
Next: Test a given array element-wise for finiteness (not infinity or not a Number).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/numpy/basic/numpy-basic-exercise-4.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics