NumPy: Test a given array element-wise for finiteness (not infinity or not a Number)
Test Finiteness of Elements
Write a NumPy program to test a given array element-wise for finiteness (not infinity or not a number).
This problem involves using NumPy to check the finiteness of each element in an array. The task is to write a program that tests each element of a given NumPy array to determine if it is finite (i.e., not infinity or NaN). This is useful for validating datasets, ensuring numerical stability, and preprocessing data before performing mathematical operations. The program demonstrates how to use NumPy's capabilities for handling special numerical values effectively.
Sample Solution :
Python Code :
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a NumPy array 'a' containing elements 1, 0, NaN (Not a Number), and infinity
a = np.array([1, 0, np.nan, np.inf])
# Printing the original array 'a'
print("Original array")
print(a)
# Checking the given array 'a' element-wise for finiteness and printing the result
print("Test a given array element-wise for finiteness :")
print(np.isfinite(a))
Output:
Original array [ 1. 0. nan inf] Test a given array element-wise for finiteness : [ True True False False]
Explanation:
In the above example -
np.isfinite() function is used to test element-wise if the values in the array 'a' are finite. The function returns a boolean array of the same shape as 'a', with True for finite values (1 and 0) and False for non-finite values (NaN and Inf).
Visual Presentation:
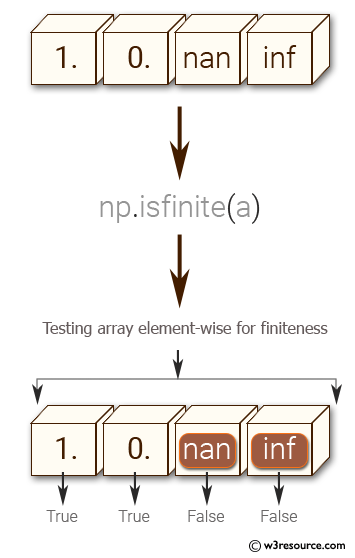
For more Practice: Solve these Related Problems:
- Check finiteness for a multi-dimensional array containing inf and NaN values.
- Filter out non-finite elements from an array and return only the finite ones.
- Apply np.isfinite on a random array and count how many elements are finite.
- Combine np.isfinite with logical operators to detect near-infinite values.
Go to:
Previous: Test if any of the elements of a given array is non-zero.
Next: Test element-wise for positive or negative infinity.
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.