NumPy: Compute the inner product of two given vectors
Compute Inner Product of Vectors
Write a NumPy program to compute the inner product of two given vectors.
This problem involves writing a NumPy program to compute the inner product of two given vectors. The inner product, also known as the dot product, involves multiplying the corresponding elements of the vectors and summing the results. By utilizing NumPy's array manipulation capabilities, particularly the dot function, the program efficiently calculates the inner product, providing a scalar value representing the measure of similarity or projection of one vector onto another.
Sample Solution :
Python Code :
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating NumPy arrays 'x' and 'y' with respective values [4, 5] and [7, 10]
x = np.array([4, 5])
y = np.array([7, 10])
# Printing a message indicating the original vectors 'x' and 'y'
print("Original vectors:")
print(x)
print(y)
# Calculating and printing the inner product of the vectors 'x' and 'y' using np.dot()
print("Inner product of said vectors:")
print(np.dot(x, y))
Output:
Original vectors: [4 5] [ 7 10] Inner product of said vectors: 78
Explanation:
The above code creates two 1D arrays 'x' and 'y' and calculates their dot product, which is then printed as the output.
x = np.array([4, 5]): This statement creates a 1D array 'x' with the elements [4, 5].
y = np.array([7, 10]): This statement creates a 1D array 'y' with the elements [7, 10].
Finally in ‘print(np.dot(x, y))’ statement np.dot() function is used to calculate the dot product of the two 1D arrays 'x' and 'y'. The dot product is computed as (4 * 7) + (5 * 10) = 28 + 50 = 78. The result, 78, is then printed to the console.
Visual Presentation:
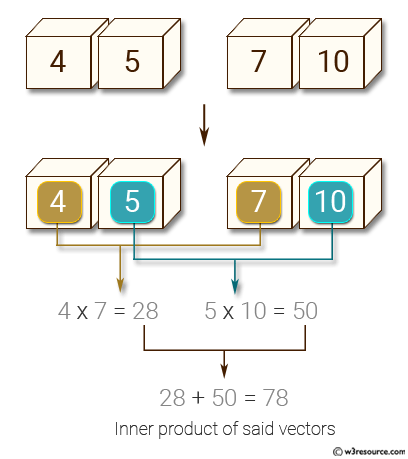
For more Practice: Solve these Related Problems:
- Calculate the inner product of two vectors using np.dot and confirm with manual multiplication.
- Compute the inner product for high-dimensional arrays after flattening them into vectors.
- Determine the inner product of two vectors and compare it with their cosine similarity.
- Implement a custom inner product function without using the built-in dot method.
Go to:
PREV : Compute Sums (All, Row, Column)
NEXT : Add Vector to Matrix Rows
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.