NumPy: Add a vector to each row of a given matrix
Add Vector to Matrix Rows
Write a NumPy program to add a vector to each row of a given matrix.
This problem involves writing a NumPy program to add a given vector to each row of a specified matrix. The task requires leveraging NumPy's broadcasting capabilities to efficiently perform the addition operation across all rows simultaneously. By adding the vector to each row, the program modifies the matrix according to the specified vector values, resulting in a new matrix with updated row elements.
Sample Solution :
Python Code :
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a NumPy array 'm' representing a matrix with 4 rows and 3 columns
m = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9], [10, 11, 12]])
# Creating a NumPy array 'v' representing a vector with 3 elements
v = np.array([1, 1, 0])
# Printing a message indicating the original vector 'v'
print("Original vector:")
print(v)
# Printing a message indicating the original matrix 'm'
print("Original matrix:")
print(m)
# Creating an empty matrix 'result' with the same shape as 'm' using np.empty_like()
result = np.empty_like(m)
# Adding the vector 'v' to each row of the matrix 'm' using a for loop
for i in range(4):
result[i, :] = m[i, :] + v
# Printing a message indicating the result after adding the vector 'v' to each row of the matrix 'm'
print("\nAfter adding the vector v to each row of the matrix m:")
print(result)
Output:
Original vector: [1 1 0] Original matrix: [[ 1 2 3] [ 4 5 6] [ 7 8 9] [10 11 12]] After adding the vector v to each row of the matrix m: [[ 2 3 3] [ 5 6 6] [ 8 9 9] [11 12 12]]
Explanation:
In the above code -
np.array([[1,2,3], [4,5,6], [7,8,9], [10, 11, 12]]) creates a 2D array and stores in the variable 'm' with the shape (4, 3) and elements [[1, 2, 3], [4, 5, 6], [7, 8, 9], [10, 11, 12]].
np.array([1, 1, 0]) creates a 1D array and stores in the variable 'v' with the elements [1, 1, 0].
result = np.empty_like(m): This line creates an empty array 'result' with the same shape and data type as the array 'm'. The contents of 'result' are not initialized, and they will be overwritten in the next step.
for i in range(4):: This line starts a loop that iterates over the 4 rows of the 2D array 'm'.
result[i, :] = m[i, :] + v: This line adds the 1D array 'v' to the current row 'i' of the 2D array 'm', and assigns the result to the corresponding row 'i' in the 'result' array.
Finally ‘print(result)’ prints the resulting 2D array after the 1D array 'v' has been added to each row of the 2D array 'm'.
Visual Presentation:
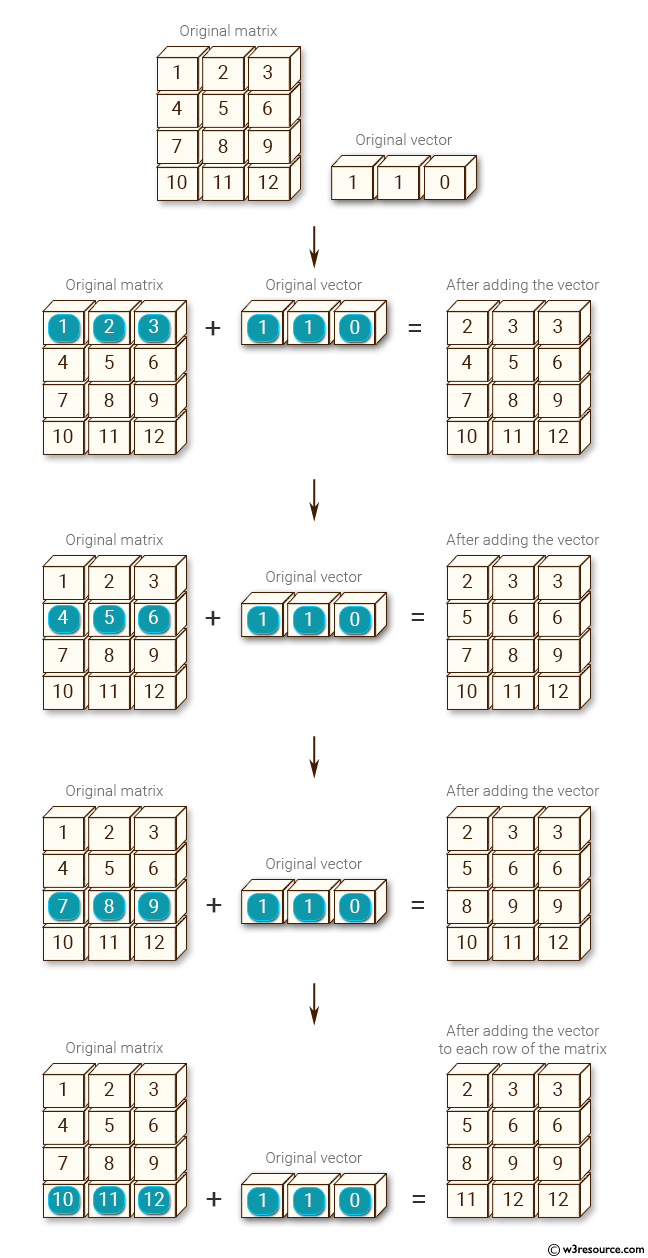
For more Practice: Solve these Related Problems:
- Add a vector to each row of a 2D array using broadcasting and verify the updated matrix.
- Concatenate a vector to every row of a matrix and then subtract another vector from it.
- Implement row-wise addition of a vector using both loops and vectorized operations.
- Add a vector to each row of a matrix and then compute the mean value of each updated row.
Go to:
PREV : Compute Inner Product of Vectors
NEXT : Save Array to Binary File
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.