NumPy: Find the memory size of a NumPy array
Memory Size of Array
Write a NumPy program to find the memory size of a NumPy array.
Pictorial Presentation:
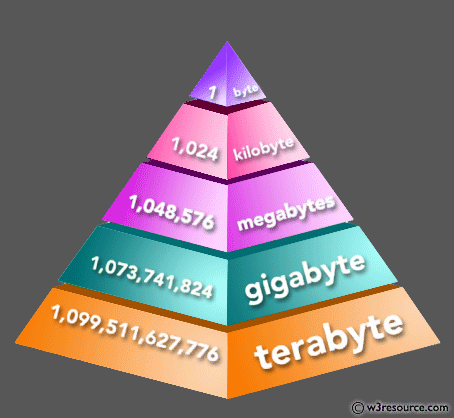
Sample Solution:
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a 4x4 array filled with zeros
n = np.zeros((4,4))
# Calculating the total memory consumed by the array and displaying it in bytes
print("%d bytes" % (n.size * n.itemsize))
Sample Output:
128 bytes
Explanation:
The above code creates a NumPy array filled with zeros and calculates its memory size in bytes.
n = np.zeros((4,4)): This statement creates a NumPy array n of shape (4, 4) filled with zeros. By default, the dtype is 'float64', meaning each element takes up 8 bytes of memory.
print("%d bytes" % (n.size * n.itemsize)): This statement calculates the total memory size of the array in bytes and prints it. The .size attribute returns the number of elements in the array (4 * 4 = 16), and the .itemsize attribute returns the memory size of one element in bytes (8 bytes for a float64). The product of these two values (16 * 8 = 128) gives the total memory size of the array in bytes.
For more Practice: Solve these Related Problems:
- Determine the total memory size of an array using its nbytes attribute and compare it to manual calculations.
- Create a function that prints the memory usage in bytes, kilobytes, and megabytes for a given array.
- Test memory size differences when altering the dtype of an array while keeping its shape constant.
- Compare memory sizes for arrays created with np.zeros versus np.empty to illustrate initialization differences.
Go to:
PREV : Save Array to Text File
NEXT : Create Zeros and Ones Array
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.