NumPy: Access an array by column
Access Array by Column
Write a NumPy program to access an array by column.
Pictorial Presentation:
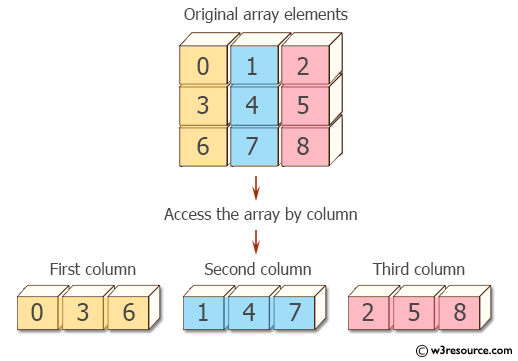
Sample Solution:
Python Code:
# Importing the NumPy library and aliasing it as 'np'
import numpy as np
# Creating a 1-dimensional array 'x' with values from 0 to 8 and reshaping it into a 3x3 array
x = np.arange(9).reshape(3, 3)
# Printing a message indicating the original array elements will be shown
print("Original array elements:")
# Printing the original array 'x' with its elements
print(x)
# Printing a message indicating that an array will be accessed by columns
print("Access an array by column:")
# Printing a message indicating the display of the first column of the array
print("First column:")
# Printing the first column of the array 'x' using slicing with ":" for rows and "0" for the first column index
print(x[:, 0])
# Printing a message indicating the display of the second column of the array
print("Second column:")
# Printing the second column of the array 'x' using slicing with ":" for rows and "1" for the second column index
print(x[:, 1])
# Printing a message indicating the display of the third column of the array
print("Third column:")
# Printing the third column of the array 'x' using slicing with ":" for rows and "2" for the third column index
print(x[:, 2])
Sample Output:
Original array elements: [[0 1 2] [3 4 5] [6 7 8]] Access an array by column: First column: [0 3 6] Second column: [1 4 7] Third column: [2 5 8]
Explanation:
In the above example -
x = np.arange(9).reshape(3,3): Create a NumPy array x using np.arange(9), which generates an array with values from 0 to 8. Then, reshape the array into a 3x3 matrix using the reshape method.
print(x[:,0]) - Print the first column of the array x. The colon : in the row position indicates selecting all rows, and the 0 in the column position indicates selecting the first column.
print(x[:,1]) - Print the second column of the array x. The colon : in the row position indicates selecting all rows, and the 1 in the column position indicates selecting the second column.
print(x[:,2]) - Print the third column of the array x. The colon : in the row position indicates selecting all rows, and the 2 in the column position indicates selecting the third column.
For more Practice: Solve these Related Problems:
- Write a NumPy program to extract a specific column from a 2D array using slicing and indexing.
- Create a function that returns any column of an array given its index and verifies the output data.
- Use advanced indexing to access multiple non-consecutive columns from an array and check the resulting shape.
- Implement a method that swaps two columns in an array and then accesses each column individually to verify changes.
Go to:
PREV : Convert NumPy Array to Python List
NEXT : Convert Float Array to Integer Array
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.