NumPy: Convert a NumPy array of float values to a NumPy array of integer values
NumPy: Array Object Exercise-82 with Solution
Convert Float Array to Integer Array
Write a NumPy program to convert a NumPy array of floating values to a numpy array of integer values.
Pictorial Presentation:
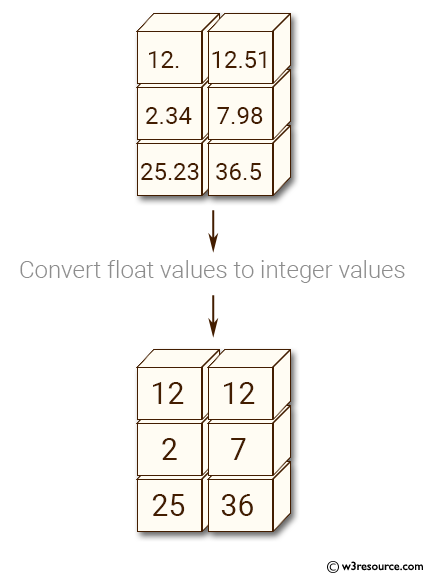
Sample Solution:
Python Code:
# Importing the NumPy library and aliasing it as 'np'
import numpy as np
# Creating a 2-dimensional array 'x' with floating-point values
x = np.array([[12.0, 12.51], [2.34, 7.98], [25.23, 36.50]])
# Printing a message indicating the original array elements will be shown
print("Original array elements:")
# Printing the original array 'x' with its elements
print(x)
# Printing a message indicating the conversion of float values to integer values
print("Convert float values to integer values:")
# Converting the floating-point values in the array 'x' to integer values using astype(int)
# Printing the array obtained after conversion
print(x.astype(int))
Sample Output:
Original array elements: [[ 12. 12.51] [ 2.34 7.98] [ 25.23 36.5 ]] Convert float values to integer values: [[12 12] [ 2 7] [25 36]]
Explanation:
In the above code –
x = np.array([[12.0, 12.51], [2.34, 7.98], [25.23, 36.50]]): This line creates a NumPy array x with the given float values. The array has a shape of (3, 2).
print(x.astype(int)): Convert the elements of the array x to integers using the astype method, and then print the resulting array. The astype method creates a new array with the int data type. The conversion from float to int results in truncating the decimal part, effectively rounding the values down to the nearest integer.
Python-Numpy Code Editor:
Previous: Write a NumPy program to access an array by column.
Next: Write a NumPy program to display NumPy array elements of floating values with given precision.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/numpy/python-numpy-exercise-82.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics