NumPy: Count the frequency of unique values in numpy array
Frequency of Distinct Values in Array
Write a NumPy program to count the frequency of distinct values in a NumPy array.
Pictorial Presentation:
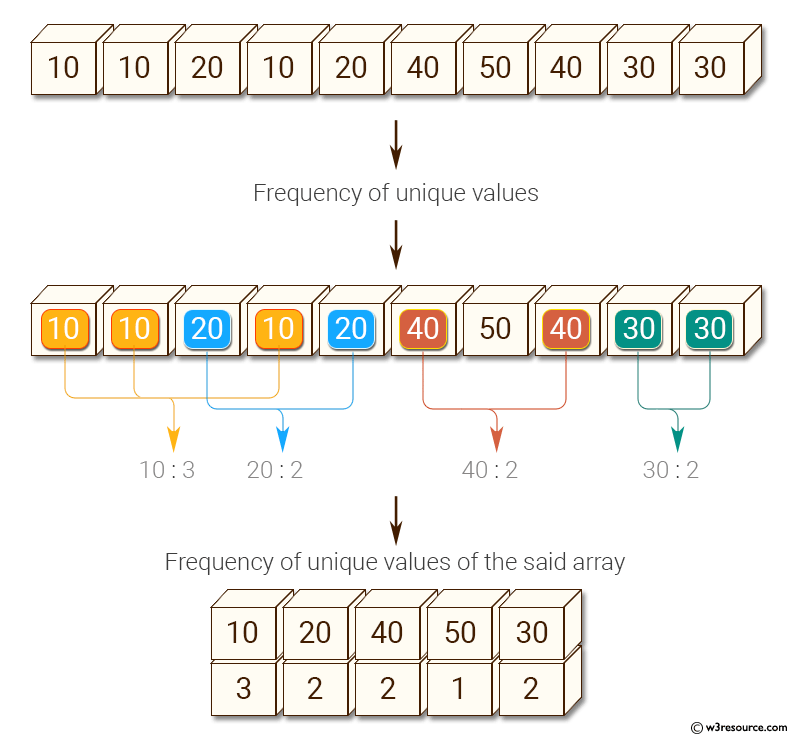
Sample Solution:
Python Code:
# Importing the NumPy library and aliasing it as 'np'
import numpy as np
# Creating a NumPy array 'a' containing integers
a = np.array([10, 10, 20, 10, 20, 20, 20, 30, 30, 50, 40, 40])
# Printing a message indicating the original array will be displayed
print("Original array:")
# Printing the original array 'a' with its elements
print(a)
# Finding unique elements and their counts in the array 'a' using np.unique() with return_counts=True
unique_elements, counts_elements = np.unique(a, return_counts=True)
# Printing a message indicating the frequency of unique values in the array
print("Frequency of unique values of the said array:")
# Creating a NumPy array from the unique elements and their respective counts
# Converting the resulting arrays into a 2D NumPy array using np.asarray()
result = np.asarray((unique_elements, counts_elements))
# Printing the array containing unique elements and their frequencies
print(result)
Sample Output:
Original array: [10 10 20 10 20 20 20 30 30 50 40 40] Frequency of unique values of the said array: [[10 20 30 40 50] [ 3 4 2 2 1]]
Explanation:
In the above code –
- a = np.array(...): Create a NumPy array 'a' containing the given integer values.
- np.unique(a, return_counts=True): Find the unique elements in the array 'a' and their counts using the np.unique function. The return_counts parameter is set to True, so the function returns two arrays: one containing the unique elements and another containing the corresponding counts of those unique elements.
- unique_elements, counts_elements = ...: Assign the returned unique elements and their counts to the variables unique_elements and counts_elements, respectively.
- np.asarray((unique_elements, counts_elements)): Combine the unique elements and their counts into a single 2D NumPy array.
- print(...): Print the resulting 2D NumPy array.
For more Practice: Solve these Related Problems:
- Write a NumPy program to count the frequency of unique elements in an array using np.unique with return_counts.
- Create a function that returns both the unique values and their corresponding frequencies from a given array.
- Test the frequency count on an array with repeated elements and verify the ordering of the output.
- Compare the results of np.bincount on an integer array with the output from np.unique to validate consistency.
Go to:
PREV : Find Magnitude of Vector
NEXT : Check if Array is Empty
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.