Concatenating NumPy Arrays Vertically
Concatenate two NumPy arrays vertically.
Sample Solution:
Python Code:
import numpy as np
# Create two NumPy arrays
array1 = np.array([[1, 2, 3],
[4, 5, 6]])
array2 = np.array([[7, 8, 9],
[10, 11, 12]])
# Concatenate arrays vertically
result_array = np.vstack((array1, array2))
# Display the result
print(result_array)
Output:
[[ 1 2 3] [ 4 5 6] [ 7 8 9] [10 11 12]]
Explanation:
Here's a breakdown of the above code:
- We created two NumPy arrays, "array1" and "array2", each with two rows and three columns.
- The np.vstack((array1, array2)) function concatenates the arrays vertically.
- The resulting result_array is a NumPy array with four rows and three columns.
- The result is printed, showing the vertically concatenated array.
We can also use np.concatenate() with axis=0 for vertical concatenation:
result_array = np.concatenate((array1, array2), axis=0)
Flowchart:
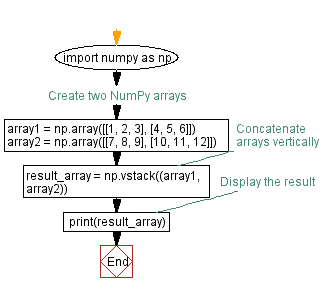
Python Code Editor:
Previous: Reshaping a 1D NumPy array into a 2D array.
Next: Matrix Multiplication with NumPy.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.