Matrix Multiplication with NumPy
Perform matrix multiplication using NumPy.
Sample Solution:
Python Code:
import numpy as np
# Create two matrices
matrix1 = np.array([[1, 2, 3],
[4, 5, 6]])
matrix2 = np.array([[7, 8],
[9, -10],
[11, 12]])
# Perform matrix multiplication using numpy.dot()
result_matrix = np.dot(matrix1, matrix2)
# Alternatively, you can use the @ operator
# result_matrix = matrix1 @ matrix2
# Display the result
print(result_matrix)
Output:
[[ 58 24] [139 54]]
Explanation:
Here's a breakdown of the above code:
- We create two matrices, "matrix1" and "matrix2", where the number of columns in "matrix1" matches the number of rows in "matrix2".
- The np.dot(matrix1, matrix2) function (or the matrix1 @ matrix2 operation) performs matrix multiplication.
- The resulting "result_matrix" is the product of the two matrices.
- The result is printed, showing the matrix multiplication result.
Flowchart:
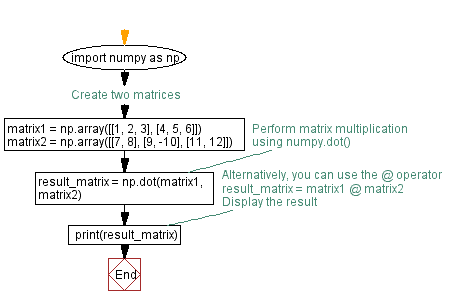
Python Code Editor:
Previous: Concatenating NumPy Arrays Vertically.
Next: Cumulative sum of a NumPy array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.