Python pprint Exercise - Print a dictionary, nested dictionary using the pprint module
1. Pprint Nested Structures
Write a Python program to print a dictionary, nested dictionary, list of dictionaries of dictionaries using the pprint module.
Sample Solution:
Python Code:
import pprint
print("Print dictionary:")
netehan_dict = {'Name': 'Metehan Christel', 'Age': 22, 'City': 'London', 'Country': 'United Kingdom'}
pprint.pprint(netehan_dict)
print("\n")
pprint.pprint(dict([('sape', 4139), ('guido', 4127), ('jack', 4098)]))
print("\nPrint nested dictionary:")
student = {1: {'name': 'Shauna Mathews', 'age': '12'},
2: {'name': 'Alexandra Powell', 'age': '11'},
3: {'name': 'Aled Carney', 'age': '11'},
4: {'name': 'Dennis Walker', 'age': '10'},
5: {'name': 'Leia Thomas', 'age': '12'}}
pprint.pprint(student)
print("\nList of dictionaries of dictionaries:")
list_of_dicts = [
{
"dict1": {
"key1": "val1",
"key2": "val2"
}
},
{
"dict2": {
"key3": "val3",
"key4": "val4"
}
}
]
pprint.pprint(list_of_dicts)
Sample Output:
Print dictionary: {'Age': 22, 'City': 'London', 'Country': 'United Kingdom', 'Name': 'Metehan Christel'} {'guido': 4127, 'jack': 4098, 'sape': 4139} Print nested dictionary: {1: {'age': '12', 'name': 'Shauna Mathews'}, 2: {'age': '11', 'name': 'Alexandra Powell'}, 3: {'age': '11', 'name': 'Aled Carney'}, 4: {'age': '10', 'name': 'Dennis Walker'}, 5: {'age': '12', 'name': 'Leia Thomas'}} List of dictionaries of dictionaries: [{'dict1': {'key1': 'val1', 'key2': 'val2'}}, {'dict2': {'key3': 'val3', 'key4': 'val4'}}]
Flowchart:
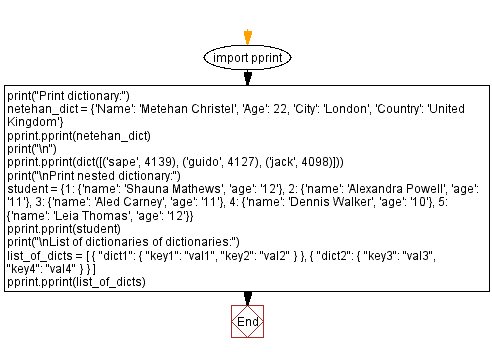
For more Practice: Solve these Related Problems:
- Write a Python program to pretty-print a complex nested dictionary containing lists and other dictionaries using pprint, ensuring that the output is readable and well-indented.
- Write a Python function that takes a list of dictionaries (each containing nested dictionaries) and prints the entire structure using pprint with default settings.
- Write a Python script that creates a nested data structure (dictionary within a dictionary with lists as values) and uses pprint to display it, then modify a key’s value and print the updated structure.
- Write a Python program to pretty-print a nested dictionary that contains both Unicode and numeric data, ensuring proper alignment and formatting using pprint.
Go to:
Previous: Python pprint Exercise Home.
Next: Sort the keys of a dictionary before printing it using the pprint module.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.