Python pprint Exercise - Sort the keys of a dictionary before printing it using the pprint module
Python pprint: Exercise-2 with Solution
Write a Python program to sort the keys of a dictionary before printing it using the pprint module.
Sample Solution:
Python Code:
import pprint
colors = {
'Red': 10,
'Green': 20,
'White': 30,
'Black': 40,
'Blue': 50,
'Orange': 70
}
print("Sort the keys of a dictionary before printing it:")
pprint.pprint(colors, sort_dicts=True)
nested_dict = {
'd1': {
'a': 1,
'c': 2,
'b': 3
},
'd2': {
'f': 4,
'e': 5,
'd': 6
},
'd3': {
'g': 7,
'h': 8,
'i': 9
}
}
print("\nSort the keys of a nested dictionary before printing it:")
pprint.pprint(nested_dict, sort_dicts=True)
Sample Output:
Sort the keys of a dictionary before printing it: {'Black': 40, 'Blue': 50, 'Green': 20, 'Orange': 70, 'Red': 10, 'White': 30} Sort the keys of a nested dictionary before printing it: {'d1': {'a': 1, 'b': 3, 'c': 2}, 'd2': {'d': 6, 'e': 5, 'f': 4}, 'd3': {'g': 7, 'h': 8, 'i': 9}}
Flowchart:
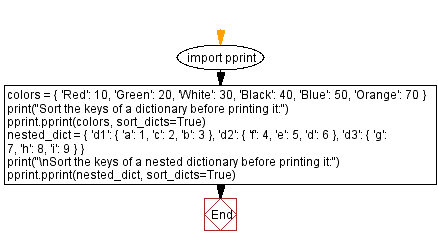
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Print a dictionary, nested dictionary using the pprint module.
Next: Specify the width of the output while printing a list, dictionary.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/pprint/python-pprint-exercise-2.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics