Python pprint Exercise - Specify the width of the output while printing a list, dictionary
Python pprint: Exercise-3 with Solution
Write a Python program that specifies the width of the output while printing a list, dictionary using the pprint module.
"width" (default 80) specifies the desired maximum number of characters per line in the output. If a structure cannot be formatted within the width constraint, a best effort will be made.
Sample Solution:
Python Code:
import pprint
colors = ["Red", "Green", "Blue", "White", "Pink"]
print("List with specific width:")
pp = pprint.PrettyPrinter(width=20)
pp.pprint(colors)
print("\nDictionary with specific width:")
nested_dict = {
'd1': {
'a': 2,
'c': 1,
'b': 3
},
'd2': {
'f': 3,
'e': 4,
'd': 6
},
'd3': {
'g': 17,
'h': 18,
'i': 9
}
}
pp.pprint(nested_dict)
Sample Output:
List with specific width: ['Red', 'Green', 'Blue', 'White', 'Pink'] Dictionary with specific width: {'d1': {'a': 2, 'b': 3, 'c': 1}, 'd2': {'d': 6, 'e': 4, 'f': 3}, 'd3': {'g': 17, 'h': 18, 'i': 9}}
Flowchart:
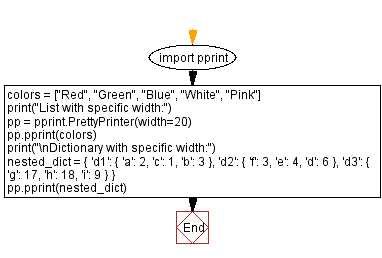
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Sort the keys of a dictionary before printing it using the pprint module.
Next: Specify the indentation while printing a nested dictionary.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics