Python pprint Exercise - Specify the indentation while printing a nested dictionary
4. Pprint with Custom Indentation
Write a Python program that specifies the indentation while printing a nested dictionary using the pprint module.
"indent" (default 1) specifies the amount of indentation added for each nesting level.
Sample Solution:
Python Code:
import pprint
print("\nNested dictionary with specific indent:")
nested_dict = {
's1': {
'a': 2,
'c': 1,
'b': 3
},
's2': {
'f': 3,
'e': 4,
'd': 6
},
's3': {
'g': 17,
'h': 18,
'i': 9
},
's4': {
'a': 7,
'j': 8,
'k': 19
}
}
pp = pprint.PrettyPrinter(indent=4)
print("\nIndent = 4")
pp.pprint(nested_dict)
print("\nIndent = 15")
pp = pprint.PrettyPrinter(indent=15)
pp.pprint(nested_dict)
Sample Output:
Nested dictionary with specific indent: Indent = 4 { 's1': {'a': 2, 'b': 3, 'c': 1}, 's2': {'d': 6, 'e': 4, 'f': 3}, 's3': {'g': 17, 'h': 18, 'i': 9}, 's4': {'a': 7, 'j': 8, 'k': 19}} Indent = 15 { 's1': {'a': 2, 'b': 3, 'c': 1}, 's2': {'d': 6, 'e': 4, 'f': 3}, 's3': {'g': 17, 'h': 18, 'i': 9}, 's4': {'a': 7, 'j': 8, 'k': 19}}
Flowchart:
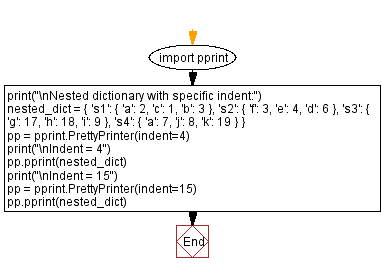
For more Practice: Solve these Related Problems:
- Write a Python program to create a deeply nested dictionary and pretty-print it using pprint with an indentation level of 4 spaces.
- Write a Python function that pretty-prints a nested data structure with a user-defined indent value and then tests it with varying levels of indentation.
- Write a Python script to generate a nested dictionary representing organizational data and print it using pprint with a custom indent to enhance readability.
- Write a Python program to modify the default indent of pprint and print a complex nested dictionary to compare the visual differences between indent=1 and indent=4.
Go to:
Previous: Specify the width of the output while printing a list, dictionary.
Next: Fetch information about a project.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.