Python pprint Exercise - Fetch information about a project
Python pprint: Exercise-5 with Solution
Write a Python program to fetch information about a project (from PyPI) using pprint() function.
Sample Solution:
Python Code:
import json
import pprint
from urllib.request import urlopen
with urlopen('https://pypi.org/pypi/scriptgen/json') as resp:
project_info = json.load(resp)['info']
pprint.pprint(project_info)
Sample Output:
{'author': 'Elmer Nocon, fopoon', 'author_email': '[email protected]', 'bugtrack_url': None, 'classifiers': ['Development Status :: 1 - Planning', 'License :: OSI Approved :: MIT License', 'Operating System :: MacOS :: MacOS X', 'Operating System :: Microsoft :: Windows :: Windows 10', 'Operating System :: Unix', 'Programming Language :: Python :: 3', 'Programming Language :: Python :: 3 :: Only', 'Programming Language :: Python :: 3.7'], 'description': '[//]: # (Auto-generated: 2021-01-13T09:44:07.865904)\n' '\n' '# scriptgen\n' '\n' '[//]: # (badges)\n' '[](https://github.com/Fopoon/scriptgen)\n' '[](https://github.com/Fopoon/scriptgen/releases)\n' '[](https://pypi.org/project/scriptgen/#history)\n' '\n' '\n' '[](LICENSE.txt)\n' '\n' '[](https://pepy.tech/project/scriptgen)\n' '\n' 'A collection of script generation helpers and templates.\n' '\n' '## Installation\n' '\n' '```sh\n' 'pip install scriptgen\n' '```\n' '\n' '## Usage\n' '\n' '```python\n' 'from scriptgen import StringBuilder\n' '\n' '\n' 'if __name__ == "__main__":\n' ' # Create a StringBuilder instance.\n' ' sb = StringBuilder()\n' '\n' ' sb.wt("Hello ") # write text "Hello "\n' ' sb.wl("World!") # write line "World!\\n"\n' '\n' ' print(str(sb))\n' '```\n' '\n' '```\n' 'Hello World!\n' '\n' '```\n' '\n' '
\n' '\n' '```python\n' 'from scriptgen import StringBuilder\n' '\n' 'from scriptgen.templates.csharp import \\\n' ' csharp_usings, \\\n' ' csharp_namespace, \\\n' ' csharp_class, \\\n' ' csharp_method\n' '\n' '\n' 'if __name__ == "__main__":\n' ' # Create a StringBuilder instance.\n' ' sb = StringBuilder()\n' '\n' ' # Write using statements.\n' ' sb.wb(csharp_usings(\n' ' "System"\n' ' ))\n' '\n' ' # Add a new line after the using statements.\n' ' sb.nl()\n' '\n' ' # Create a namespace StringBuilder instance.\n' ' ns = csharp_namespace("Sample")\n' '\n' ' # Create a class StringBuilder instance.\n' ' c = csharp_class(\n' ' class_name="Program",\n' ' access_modifier="public"\n' ' )\n' '\n' ' # Create a method StringBuilder instance.\n' ' m = csharp_method(\n' ' method_name="Main",\n' ' access_modifier="public static",\n' ' return_type="int"\n' ' )\n' '\n' ' # Write the following lines inside the method.\n' ' m.wl(\'Console.WriteLine("Hello World!");\')\n' ' m.wl("return 0;")\n' '\n' ' c.wb(m) # Write the method inside the class.\n' ' ns.wb(c) # Write the class inside the namespace.\n' ' sb.wb(ns) # Write the namespace.\n' '\n' ' print(str(sb))\n' '\n' '```\n' '\n' '```csharp\n' 'using System;\n' '\n' 'namespace Sample\n' '{\n' ' public class Program\n' ' {\n' ' public static int Main()\n' ' {\n' ' Console.WriteLine("Hello World!");\n' ' return 0;\n' ' }\n' ' }\n' '}\n' '\n' '```\n' '\n' '
\n' '\n' '```python\n' 'from scriptgen import StringBuilder\n' '\n' 'from scriptgen.templates.csharp import \\\n' ' csharp_autogen, \\\n' ' csharp_namespace, \\\n' ' csharp_class, \\\n' ' csharp_region\n' '\n' '\n' 'if __name__ == "__main__":\n' ' # Get version from arguments..\n' ' # i.e. python script.py -major 0 -minor 0 -patch 1\n' ' major: int = 0\n' ' minor: int = 0\n' ' patch: int = 1\n' '\n' ' # Create a StringBuilder instance.\n' ' sb = StringBuilder()\n' '\n' ' # Write timestamp.\n' ' sb.wb(csharp_autogen())\n' '\n' ' # Add a new line after the using statements.\n' ' sb.nl()\n' '\n' ' # Create a namespace StringBuilder instance.\n' ' ns = csharp_namespace("Sample")\n' '\n' ' # Create a class StringBuilder instance.\n' ' c = csharp_class(\n' ' class_name="BuildInfo",\n' ' access_modifier="public static partial"\n' ' )\n' '\n' ' # Create a "Constants" region StringBuilder instance.\n' ' r = csharp_region("Constants")\n' '\n' ' # Write the following lines inside the "Constants" ' 'region.\n' ' r.wl(f"public const int MAJOR = {major};")\n' ' r.nl()\n' ' r.wl(f"public const int MINOR = {minor};")\n' ' r.nl()\n' ' r.wl(f"public const int PATCH = {patch};")\n' '\n' ' c.wb(r) # Write the region inside the class.\n' ' ns.wb(c) # Write the class inside the namespace.\n' ' sb.wb(ns) # Write the namespace.\n' '\n' ' print(str(sb))\n' '\n' '```\n' '\n' '```csharp\n' '// Auto-generated: 2020-03-15T04:20:47.909851\n' '\n' 'namespace Sample\n' '{\n' ' public static partial class BuildInfo\n' ' {\n' ' #region Constants\n' '\n' ' public const int MAJOR = 0;\n' '\n' ' public const int MINOR = 0;\n' '\n' ' public const int PATCH = 1;\n' '\n' ' #endregion Constants\n' ' }\n' '}\n' '\n' '```\n' '\n' '
\n' '\n' 'Look at this [script](tools/gen_docs.py) to see its practical ' 'use case.\n' '\n' '## Contribution\n' '\n' 'Suggestions and contributions are always welcome.\n' 'Make sure to read the [Contribution ' 'Guidelines](CONTRIBUTING.md) file for more information before ' 'submitting a `pull request`.\n' '\n' '## License\n' '\n' '`scriptgen` is released under the MIT License. See the ' '[LICENSE](LICENSE.txt) file for details.\n' '\n' '\n' '# Changelog\n' '\n' '## v0.0.5\n' '- Fixed multiple new lines in Windows.\n' '- Added more C# templates.\n' '\n' '## v0.0.4\n' '\n' '- Added XML templates.\n' '\n' '## v0.0.3\n' '\n' '- Added index parameter in filter_func.\n' '- Changed from current working path to file directory path in ' '`gen_docs.py` script.\n' '\n' '## v0.0.2\n' '\n' '- Added default values for the builder classes.\n' '- Added optional parameters.\n' '- Exposed `CSharpBlockBuilder` class in ' '`scriptgen.templates.csharp` package.\n' '- Bug fixes.\n' '\n' '## v0.0.1\n' '\n' '- Added `IndentType`, `StringBuilder`, and `BlockBuilder` ' 'classes.\n' '- Added `diff_lines`, `diff_text`, `interpolate_text`, ' '`timestamp`, and `write_text_file` utility methods.\n' '- Added C# and Markdown templates.\n' '- Added tests for the utility methods, C# templates, and ' 'Markdown templates.\n' '- Added `gen_docs.py` script and template files.\n' '\n', 'description_content_type': 'text/markdown', 'docs_url': None, 'download_url': 'https://pypi.org/project/scriptgen/', 'downloads': {'last_day': -1, 'last_month': -1, 'last_week': -1}, 'home_page': 'https://github.com/Fopoon/scriptgen', 'keywords': '', 'license': 'MIT', 'maintainer': '', 'maintainer_email': '', 'name': 'scriptgen', 'package_url': 'https://pypi.org/project/scriptgen/', 'platform': 'Any', 'project_url': 'https://pypi.org/project/scriptgen/', 'project_urls': {'Download': 'https://pypi.org/project/scriptgen/', 'Homepage': 'https://github.com/Fopoon/scriptgen'}, 'release_url': 'https://pypi.org/project/scriptgen/0.0.5/', 'requires_dist': None, 'requires_python': '', 'summary': 'A collection of script generation helpers and templates.', 'version': '0.0.5', 'yanked': False, 'yanked_reason': None}
Flowchart:
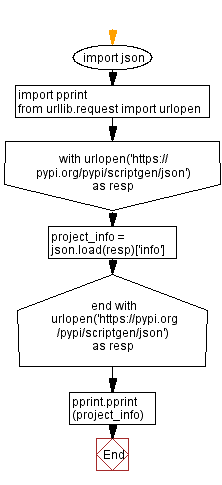
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Specify the indentation while printing a nested dictionary.
Next: Get project information limiting the results to a certain level.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics