Python pprint Exercise - Get project information limiting the results to a certain level
Python pprint: Exercise-6 with Solution
Write a Python program to fetch information about a project (i.e. PyPI) using pprint() function. Limit the result to a certain level and specify the width of the content.
Sample Solution:
Python Code:
import json
import pprint
from urllib.request import urlopen
with urlopen('https://pypi.org/pypi/aioxmpp/json') as resp:
project_info = json.load(resp)['info']
pprint.pprint(project_info, depth = 1, width = 160)
Sample Output:
{'author': 'Jonas Schäfer', 'author_email': '[email protected]', 'bugtrack_url': None, 'classifiers': [...], 'description': '``aioxmpp``\n' '###########\n' '\n' '.. image:: https://travis-ci.org/horazont/aioxmpp.svg?branch=devel\n' ' :target: https://travis-ci.org/horazont/aioxmpp\n' '\n' '.. image:: https://coveralls.io/repos/github/horazont/aioxmpp/badge.svg?branch=devel\n' ' :target: https://coveralls.io/github/horazont/aioxmpp?branch=devel\n' '\n' '.. image:: https://img.shields.io/pypi/v/aioxmpp.svg\n' ' :target: https://pypi.python.org/pypi/aioxmpp/\n' '\n' '... is a pure-python XMPP library using the `asyncio`_ standard library module from Python 3.4 (and `available as a third-party module to ' 'Python 3.3`__).\n' '\n' '.. _asyncio: https://docs.python.org/3/library/asyncio.html\n' '__ https://code.google.com/p/tulip/\n' '\n' '.. remember to update the feature list in the docs\n' '\n' 'Features\n' '========\n' '\n' '* Native `Stream Management (XEP-0198)\n' ' <https://xmpp.org/extensions/xep-0198.html>`_ support for robustness against\n' ' transient network failures (such as switching between wireless and wired\n' ' networks).\n' '\n' '* Powerful declarative-style definition of XEP-based and custom protocols. Most\n' ' of the time, you will not get in contact with raw XML or character data, even\n' ' when implementing a new protocol.\n' '\n' '* Secure by default: TLS is required by default, as well as certificate\n' ' validation. Certificate or public key pinning can be used, if needed.\n' '\n' '* Support for `RFC 6121 (Instant Messaging and Presence)\n' ' <https://tools.ietf.org/html/rfc6121>`_ roster and presence management, along\n' ' with `XEP-0045 (Multi-User Chats)\n' ' <https://xmpp.org/extensions/xep-0045.html>`_ for your human-to-human needs.\n' '\n' '* Support for `XEP-0060 (Publish-Subscribe)\n' ' <https://xmpp.org/extensions/xep-0060.html>`_ and `XEP-0050 (Ad-Hoc Commands)\n' ' <https://xmpp.org/extensions/xep-0050.html>`_ for your machine-to-machine\n' ' needs.\n' '\n' '* Several other XEPs, such as `XEP-0115\n' ' <https://xmpp.org/extensions/xep-0115.html>`_ (including native support for\n' ' the reading and writing the `capsdb <https://github.com/xnyhps/capsdb>`_) and\n' ' `XEP-0131 <https://xmpp.org/extensions/xep-0131.html>`_.\n' '\n' '* APIs suitable for both one-shot scripts and long-running multi-account\n' ' clients.\n' '\n' '* Well-tested and modular codebase: aioxmpp is developed in test-driven\n' ' style and in addition to that, many modules are automatedly tested against\n' ' `Prosody <https://prosody.im/>`_ and `ejabberd <https://www.ejabberd.im/>`_,\n' ' two popular XMPP servers.\n' '\n' '\n' 'There is more and there’s yet more to come! Check out the list of supported XEPs\n' 'in the `official documentation`_ and `open GitHub issues tagged as enhancement\n' '<https://github.com/horazont/aioxmpp/issues?q=is%3Aissue+is%3Aopen+label%3Aenhancement>`_\n' 'for things which are planned and read on below on how to contribute.\n' '\n' 'Documentation\n' '=============\n' '\n' 'The ``aioxmpp`` API is thoroughly documented using Sphinx. Check out the `official documentation`_ for a `quick start`_ and the `API ' 'reference`_.\n' '\n' 'Dependencies\n' '============\n' '\n' '* Python ≥ 3.4 (or Python = 3.3 with tulip and enum34)\n' '* DNSPython\n' '* lxml\n' '* `sortedcollections`__\n' '\n' ' __ https://pypi.python.org/pypi/sortedcollections\n' '\n' '* `tzlocal`__ (for i18n support)\n' '\n' ' __ https://pypi.python.org/pypi/tzlocal\n' '\n' '* `pyOpenSSL`__\n' '\n' ' __ https://pypi.python.org/pypi/pyOpenSSL\n' '\n' '* `pyasn1`_ and `pyasn1_modules`__\n' '\n' ' .. _pyasn1: https://pypi.python.org/pypi/pyasn1\n' ' __ https://pypi.python.org/pypi/pyasn1-modules\n' '\n' '* `aiosasl`__ (≥ 0.3 for ``ANONYMOUS`` support)\n' '\n' ' __ https://pypi.python.org/pypi/aiosasl\n' '\n' '* `multidict`__\n' '\n' ' __ https://pypi.python.org/pypi/multidict\n' '\n' '* `aioopenssl`__\n' '\n' ' __ https://github.com/horazont/aioopenssl\n' '\n' '* `typing`__ (Python < 3.5 only)\n' '\n' ' __ https://pypi.python.org/pypi/typing\n' '\n' 'Contributing\n' '============\n' '\n' 'If you consider contributing to aioxmpp, you can do so, even without a GitHub\n' 'account. There are several ways to get in touch with the aioxmpp developer(s):\n' '\n' '* `The development mailing list\n' ' <https://lists.zombofant.net/cgi-bin/mailman/listinfo/aioxmpp-devel>`_. Feel\n' ' free to subscribe and post, but be polite and adhere to the `Netiquette\n' ' (RFC 1855) <https://tools.ietf.org/html/rfc1855>`_. Pull requests posted to\n' ' the mailing list are also welcome!\n' '\n' '* The development MUC at ``[email protected]``. Pull requests\n' ' announced in the MUC are also welcome! Note that the MUC is set persistent,\n' ' but nevertheless there may not always be people around. If in doubt, use the\n' ' mailing list instead.\n' '\n' '* Open or comment on an issue or post a pull request on `GitHub\n' ' <https://github.com/horazont/aioxmpp/issues>`_.\n' '\n' 'No idea what to do, but still want to get your hands dirty? Check out the list\n' "of `'help wanted' issues on GitHub\n" '<https://github.com/horazont/aioxmpp/issues?q=is%3Aissue+is%3Aopen+label%3A%22help+wanted%22>`_\n' "or ask in the MUC or on the mailing list. The issues tagged as 'help wanted' are\n" 'usually of narrow scope, aimed at beginners.\n' '\n' 'Be sure to read the ``docs/CONTRIBUTING.rst`` for some hints on how to\n' 'author your contribution.\n' '\n' 'Security issues\n' '---------------\n' '\n' 'If you believe that a bug you found in aioxmpp has security implications,\n' 'you are welcome to notify me privately. To do so, send a mail to `Jonas Schäfer\n' '<mailto:[email protected]>`_, encrypted using the GPG public key\n' '0xE5EDE5AC679E300F (Fingerprint AA5A 78FF 508D 8CF4 F355 F682 E5ED E5AC 679E\n' '300F).\n' '\n' 'If you prefer to disclose security issues immediately, you can do so at any of\n' 'the places listed above.\n' '\n' 'More details can be found in the `SECURITY.md <SECURITY.md>`_ file.\n' '\n' 'Change log\n' '==========\n' '\n' 'The `change log`_ is included in the `official documentation`_.\n' '\n' '.. _change log: https://docs.zombofant.net/aioxmpp/0.13/api/changelog.html\n' '.. _official documentation: https://docs.zombofant.net/aioxmpp/0.13/\n' '.. _quick start: https://docs.zombofant.net/aioxmpp/0.13/user-guide/quickstart.html\n' '.. _API reference: https://docs.zombofant.net/aioxmpp/0.13/api/index.html\n', 'description_content_type': '', 'docs_url': None, 'download_url': '', 'downloads': {...}, 'home_page': 'https://github.com/horazont/aioxmpp', 'keywords': 'asyncio xmpp library', 'license': 'LGPLv3+', 'maintainer': '', 'maintainer_email': '', 'name': 'aioxmpp', 'package_url': 'https://pypi.org/project/aioxmpp/', 'platform': None, 'project_url': 'https://pypi.org/project/aioxmpp/', 'project_urls': {...}, 'release_url': 'https://pypi.org/project/aioxmpp/0.13.3/', 'requires_dist': None, 'requires_python': '', 'summary': 'Pure-python XMPP library for asyncio', 'version': '0.13.3', 'yanked': False, 'yanked_reason': None}
Flowchart:
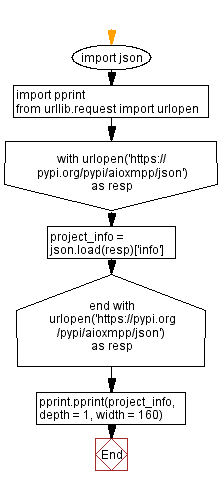
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Specify the indentation while printing a nested dictionary.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/pprint/python-pprint-exercise-6.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics