Python: Find a string consisting of the non-negative integers up to n inclusive
Python Programming Puzzles: Exercise-17 with Solution
Create String of Non-Negatives to n
Write a Python program to create a string consisting of non-negative integers up to n inclusive.
Input: 4 Output: 0 1 2 3 4 Input: 15 Output: 0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
Visual Presentation:
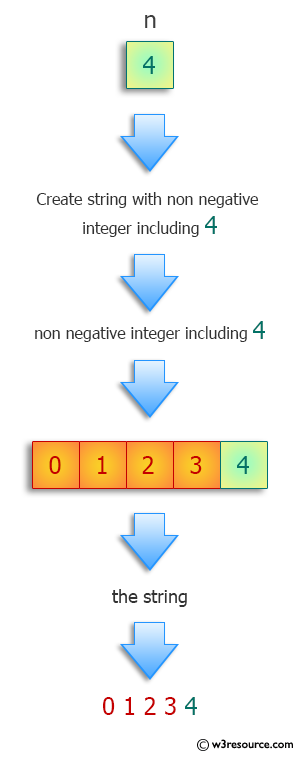
Sample Solution-1:
Python Code:
# Define a function named 'test' that takes a non-negative integer 'n' as input
def test(n):
# Use the map function to convert each integer in the range from 0 to 'n' (inclusive) to a string
# Then, use ' '.join to concatenate the strings with a space separator
return ' '.join(map(str, range(n + 1)))
# Assign a specific non-negative integer 'n' to the variable
n = 4
# Print the non-negative integer
print("Non-negative integer:")
print(n)
# Print a message indicating the operation to be performed
print("Non-negative integers up to n inclusive:")
# Print the result of the test function applied to the 'n' value
print(test(n))
# Assign a different non-negative integer 'n' to the variable
n = 15
# Print the non-negative integer
print("\nNon-negative integer:")
print(n)
# Print a message indicating the operation to be performed
print("Non-negative integers up to n inclusive:")
# Print the result of the test function applied to the updated 'n' value
print(test(n))
Sample Output:
Non-negative integer: 4 Non-negative integers up to n inclusive: 0 1 2 3 4 Non-negative integer: 15 Non-negative integers up to n inclusive: 0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
Flowchart:
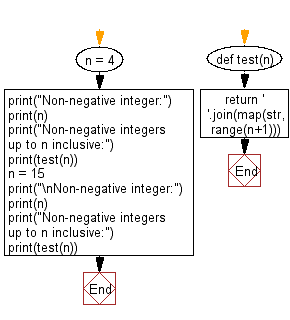
Sample Solution-2:
Python Code:
# Define a function named 'test' that takes a non-negative integer 'n' as input
def test(n):
# Use a generator expression to convert each integer in the range from 0 to 'n' (inclusive) to a string
# Then, use ' '.join to concatenate the strings with a space separator
return ' '.join(str(i) for i in range(n + 1))
# Assign a specific non-negative integer 'n' to the variable
n = 4
# Print the non-negative integer
print("Non-negative integer:")
print(n)
# Print a message indicating the operation to be performed
print("Non-negative integers up to n inclusive:")
# Print the result of the test function applied to the 'n' value
print(test(n))
# Assign a different non-negative integer 'n' to the variable
n = 15
# Print the non-negative integer
print("\nNon-negative integer:")
print(n)
# Print a message indicating the operation to be performed
print("Non-negative integers up to n inclusive:")
# Print the result of the test function applied to the updated 'n' value
print(test(n))
Sample Output:
Non-negative integer: 4 Non-negative integers up to n inclusive: 0 1 2 3 4 Non-negative integer: 15 Non-negative integers up to n inclusive: 0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
Flowchart:
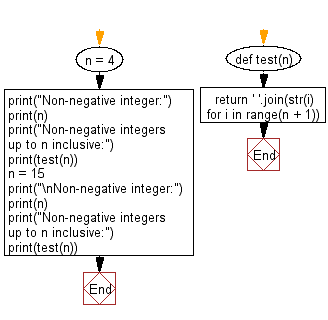
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Find the strings in a list containing a given substring.
Next: Find the indices of all occurrences of target in the uneven matrix.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/puzzles/python-programming-puzzles-17.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics