Python: Find the indices of all occurrences of target in the uneven matrix
Python Programming Puzzles: Exercise-18 with Solution
Target Indices in Uneven Matrix
An irregular/uneven matrix, or ragged matrix, is a matrix that has a different number of elements in each row. Ragged matrices are not used in linear algebra, since standard matrix transformations cannot be performed on them, but they are useful as arrays in computing.
Write a Python program to find the indices of all occurrences of target in the uneven matrix.
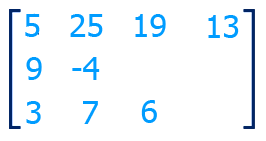
Input: [([1, 3, 2, 32, 19], [19, 2, 48, 19], [], [9, 35, 4], [3, 19]), 19] Output: [[0, 4], [1, 0], [1, 3], [4, 1]] Input: [([1, 2, 3, 2], [], [7, 9, 2, 1, 4]),2] Output: [[0, 1], [0, 3], [2, 2]]
Visual Presentation:
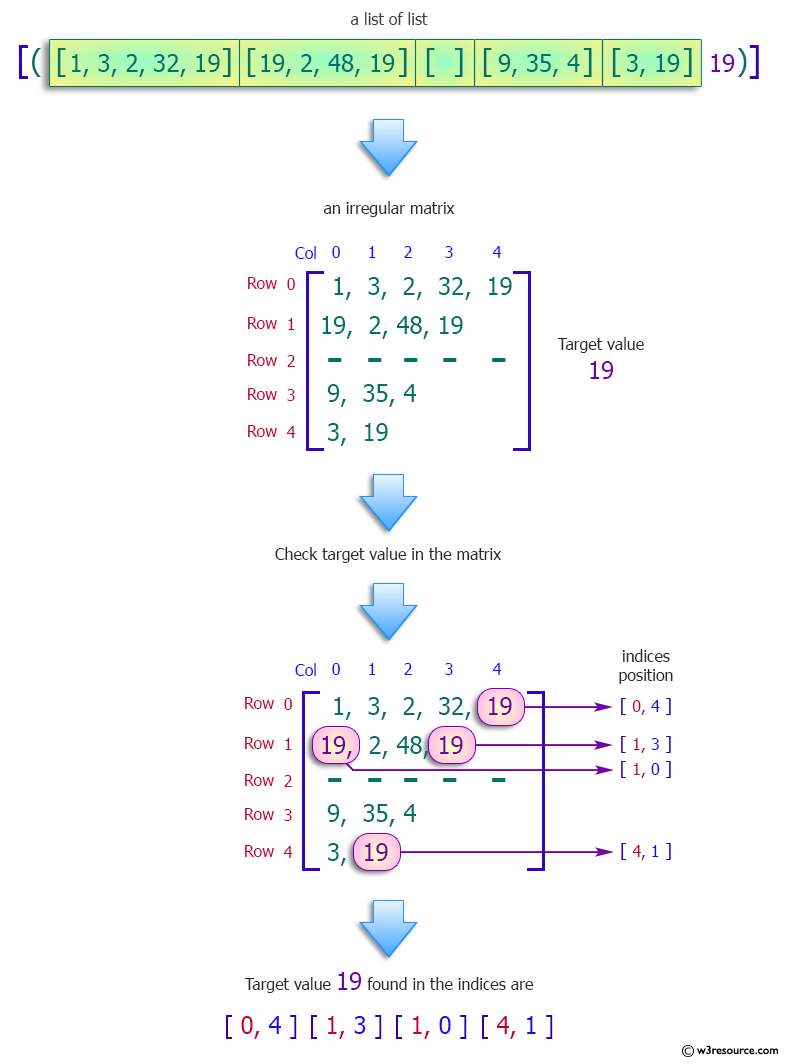
Sample Solution-1:
Python Code:
# Define a function named 'test' that takes an uneven matrix 'M' and a target value 'T' as input
def test(M, T):
# Use a nested list comprehension to generate a list of indices [i, j] for all occurrences of the target value 'T' in matrix 'M'
return [[i, j] for i, row in enumerate(M) for j, n in enumerate(row) if n == T]
# Create an uneven matrix 'M' with specific elements
M = [[1, 3, 2, 32, 19], [19, 2, 48, 19], [], [9, 35, 4], [3, 19]]
# Assign a specific target value 'T' to the variable
T = 19
# Print the matrix 'M'
print("Matrix:")
print(M)
# Print the target value 'T'
print("Target value:")
print(T)
# Print a message indicating the operation to be performed
print("Indices of all occurrences of the target value in the said uneven matrix:")
# Print the result of the test function applied to the 'M' matrix and the 'T' target value
print(test(M, T))
# Create a different uneven matrix 'M' with specific elements
M = [[1, 2, 3, 2], [], [7, 9, 2, 1, 4]]
# Assign a different target value 'T' to the variable
T = 2
# Print the matrix 'M'
print("\nMatrix:")
print(M)
# Print the target value 'T'
print("Target value:")
print(T)
# Print a message indicating the operation to be performed
print("Indices of all occurrences of the target value in the said uneven matrix:")
# Print the result of the test function applied to the updated 'M' matrix and the updated 'T' target value
print(test(M, T))
Sample Output:
Matrix: [[1, 3, 2, 32, 19], [19, 2, 48, 19], [], [9, 35, 4], [3, 19]] Target value: 19 Indices of all occurrences of the target value in the said uneven matrix: [[0, 4], [1, 0], [1, 3], [4, 1]] Matrix: [[1, 2, 3, 2], [], [7, 9, 2, 1, 4]] Target value: 2 Indices of all occurrences of the target value in the said uneven matrix: [[0, 1], [0, 3], [2, 2]]
Flowchart:
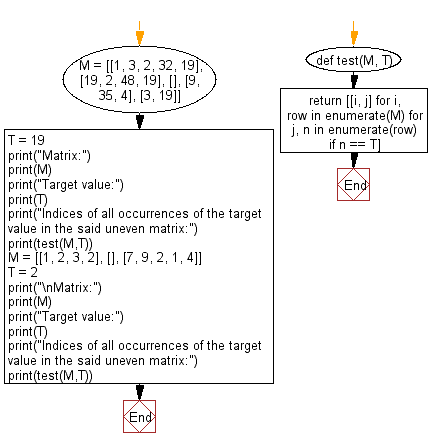
Sample Solution-2:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes an uneven matrix 'M' and a target value 'T' as input
def test(M, T):
# Initialize an empty list 'indices' to store the indices of the found elements
indices = []
# Search for the target value 'T' in the first row of the matrix 'M'
for i, num in enumerate(M[0]):
if num == T:
# Append the index [0, i] to 'indices' if the target value is found
indices.append([0, i])
# Search for the target value 'T' in the remaining rows of the matrix 'M'
for row, row_num in zip(M[1:], range(1, len(M))):
for i, num in enumerate(row):
if num == T:
# Append the index [row_num, i] to 'indices' if the target value is found in subsequent rows
indices.append([row_num, i])
# Return the list of indices of all occurrences of the target value in the uneven matrix 'M'
return indices
# Create an uneven matrix 'M' with specific elements
M = [[1, 3, 2, 32, 19], [19, 2, 48, 19], [], [9, 35, 4], [3, 19]]
# Assign a specific target value 'T' to the variable
T = 19
# Print the matrix 'M'
print("Matrix:")
print(M)
# Print the target value 'T'
print("Target value:")
print(T)
# Print a message indicating the operation to be performed
print("Indices of all occurrences of the target value in the said uneven matrix:")
# Print the result of the test function applied to the 'M' matrix and the 'T' target value
print(test(M, T))
# Create a different uneven matrix 'M' with specific elements
M = [[1, 2, 3, 2], [], [7, 9, 2, 1, 4]]
# Assign a different target value 'T' to the variable
T = 2
# Print the matrix 'M'
print("\nMatrix:")
print(M)
# Print the target value 'T'
print("Target value:")
print(T)
# Print a message indicating the operation to be performed
print("Indices of all occurrences of the target value in the said uneven matrix:")
# Print the result of the test function applied to the updated 'M' matrix and the updated 'T' target value
print(test(M, T))
Sample Output:
Matrix: [[1, 3, 2, 32, 19], [19, 2, 48, 19], [], [9, 35, 4], [3, 19]] Target value: 19 Indices of all occurrences of the target value in the said uneven matrix: [[0, 4], [1, 0], [1, 3], [4, 1]] Matrix: [[1, 2, 3, 2], [], [7, 9, 2, 1, 4]] Target value: 2 Indices of all occurrences of the target value in the said uneven matrix: [[0, 1], [0, 3], [2, 2]]
Flowchart:
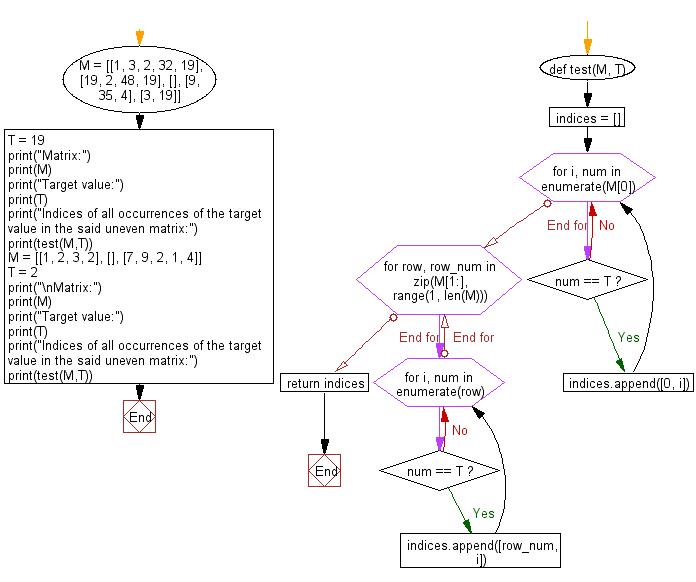
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Find a string consisting of the non-negative integers up to n inclusive.
Next: Split a string into strings if there is a space in the string, otherwise split on commas, otherwise the list of lowercase letters with odd order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/puzzles/python-programming-puzzles-18.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics