Python: Find the indices of two entries that show that the list is not in increasing order
Python Programming Puzzles: Exercise-48 with Solution
Write a Python program to find the indices of two entries that show that the list is not in increasing order. If there are no violations (they are increasing), return an empty list.
Input: [1, 2, 3, 0, 4, 5, 6] Output: [2, 3] Input: [1, 2, 3, 4, 5, 6] Output: [] Input: [1, 2, 3, 4, 6, 5, 7] Output: [4, 5] Input: [-3, -2, -3, 0, 2, 3, 4] Output: [1, 2]
Visual Presentation:
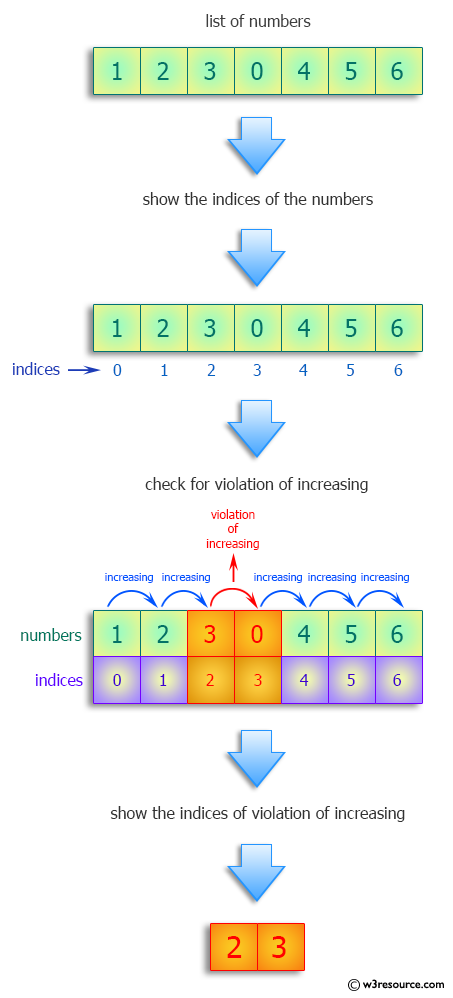
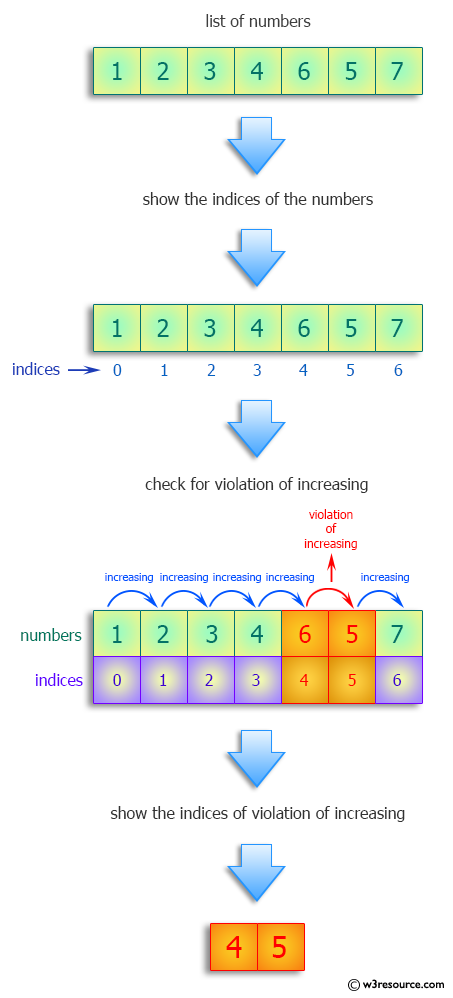
Sample Solution:
Python Code:
# Define a function named 'test' that takes a list of numbers 'nums' as input
def test(nums):
# Iterate over the indices of the list, except the last element
for i in range(len(nums) - 1):
# Check if the current element is greater than or equal to the next element
if nums[i] >= nums[i + 1]:
# Return the indices of the two entries that violate the increasing order
return [i, i + 1]
# If no violation is found, return an empty list
return []
# Assign a specific list of numbers to the variable 'nums'
nums = [1,2,3,0,4,5,6]
# Print a message indicating the original list of numbers
print("Original list:")
# Print the original list of numbers
print(nums)
# Print a message indicating the indices of two entries that violate the increasing order
print("Indices of two entries that show that the list is not in increasing order:")
# Print the result of the test function applied to 'nums'
print(test(nums))
# Assign another specific list of numbers to the variable 'nums'
nums = [1,2,3,4,5,6]
# Print a message indicating the original list of numbers
print("\nOriginal list:")
# Print the original list of numbers
print(nums)
# Print a message indicating the indices of two entries that violate the increasing order
print("Indices of two entries that show that the list is not in increasing order:")
# Print the result of the test function applied to 'nums'
print(test(nums))
# Assign another specific list of numbers to the variable 'nums'
nums = [1,2,3,4,6,5,7]
# Print a message indicating the original list of numbers
print("\nOriginal list:")
# Print the original list of numbers
print(nums)
# Print a message indicating the indices of two entries that violate the increasing order
print("Indices of two entries that show that the list is not in increasing order:")
# Print the result of the test function applied to 'nums'
print(test(nums))
# Assign another specific list of numbers to the variable 'nums'
nums = [-3,-2,-3,0,2,3,4]
# Print a message indicating the original list of numbers
print("\nOriginal list:")
# Print the original list of numbers
print(nums)
# Print a message indicating the indices of two entries that violate the increasing order
print("Indices of two entries that show that the list is not in increasing order:")
# Print the result of the test function applied to 'nums'
print(test(nums))
Sample Output:
Original list: [1, 2, 3, 0, 4, 5, 6] Indices of two entries that show that the list is not in increasing order: [2, 3] Original list: [1, 2, 3, 4, 5, 6] Indices of two entries that show that the list is not in increasing order: [] Original list: [1, 2, 3, 4, 6, 5, 7] Indices of two entries that show that the list is not in increasing order: [4, 5] Original list: [-3, -2, -3, 0, 2, 3, 4] Indices of two entries that show that the list is not in increasing order: [1, 2]
Flowchart:
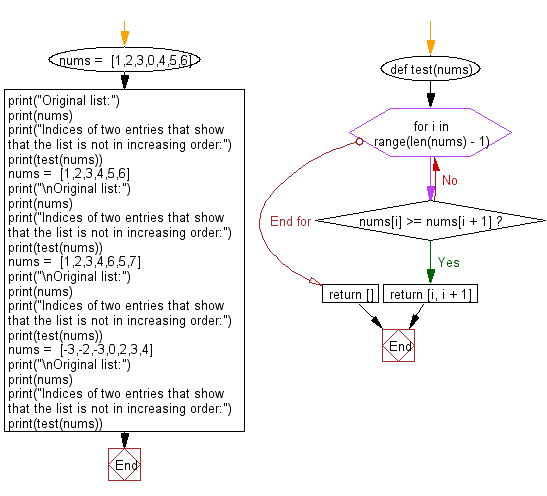
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Filter for the numbers in a list whose sum of digits is >0, where the first digit can be negative.
Next: Find the h-index, the largest positive number h such that h occurs in the sequence at least h times.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/puzzles/python-programming-puzzles-48.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics