Python: Find a palindrome of a given length containing a given string
Python Programming Puzzles: Exercise-95 with Solution
Generate Palindrome of Specific Length
Write a Python program to generate a palindrome of a given length from a string.
Input: madam , 7 Output: madaadam Input: madam , 6 Output: maddam Input: madam , 5 Output: maaaam Input: madam , 3 Output: maam Input: madam , 2 Output: mm Input: madam , 1 Output: aa
Sample Solution:
Python Code:
# License: https://bit.ly/3oLErEI
# Function to generate a palindrome of a given length from a string
def test(s, length):
s_index = 0
# Calculate the half length of the palindrome
length_half = (length - (length % 2)) // 2
ans = ""
# Build the first half of the palindrome
while len(ans) < length_half:
ans += s[s_index % len(s)]
s_index += 1
# Add a middle character if the length is odd
if length % 2 == 1:
ans += "a"
# Complete the palindrome by adding the reversed first half
return ans + ans[::-1]
# Test cases with different string and palindrome lengths
s = 'madam'
length = 7
print("String and length of the palindrome:", s, ",", length)
print("Palindrome of the said string and length:")
print(test(s, length))
s = 'madam'
length = 6
print("\nString and length of the palindrome:", s, ",", length)
print("Palindrome of the said string and length:")
print(test(s, length))
length = 5
print("\nString and length of the palindrome:", s, ",", length)
print("Palindrome of the said string and length:")
print(test(s, length))
length = 3
print("\nString and length of the palindrome:", s, ",", length)
print("Palindrome of the said string and length:")
print(test(s, length))
length = 2
print("\nString and length of the palindrome:", s, ",", length)
print("Palindrome of the said string and length:")
print(test(s, length))
length = 1
print("\nString and length of the palindrome:", s, ",", length)
print("Palindrome of the said string and length:")
print(test(s, length))
Sample Output:
String and length of the palindrome: madam , 7 Palindrome of the said string and length: madaadam String and length of the palindrome: madam , 6 Palindrome of the said string and length: maddam String and length of the palindrome: madam , 5 Palindrome of the said string and length: maaaam String and length of the palindrome: madam , 3 Palindrome of the said string and length: maam String and length of the palindrome: madam , 2 Palindrome of the said string and length: mm String and length of the palindrome: madam , 1 Palindrome of the said string and length: aa
Flowchart:
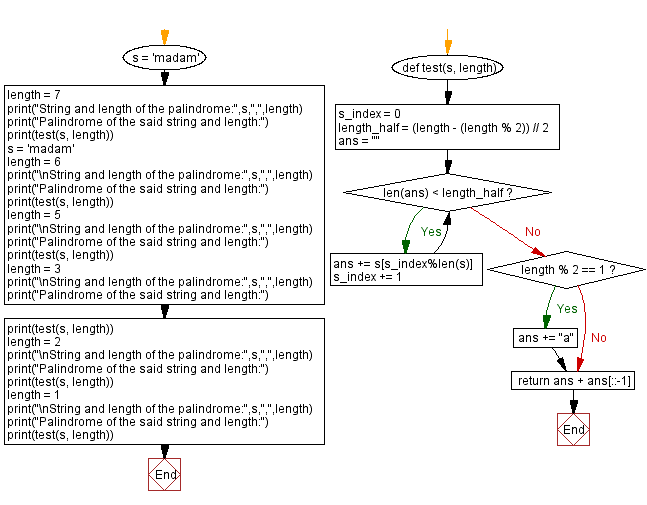
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Separate parentheses groups.
Next: Single digits in numbers sorted backwards and converted to English words.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/puzzles/python-programming-puzzles-95.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics