Python: How to check if a number is odd or even?
Even or Odd Checker
Write a Python program that determines whether a given number (accepted from the user) is even or odd, and prints an appropriate message to the user.
Pictorial Presentation of Even Numbers:
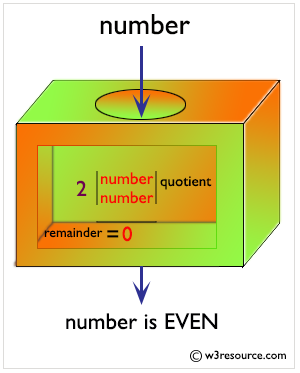
Pictorial Presentation of Odd Numbers:
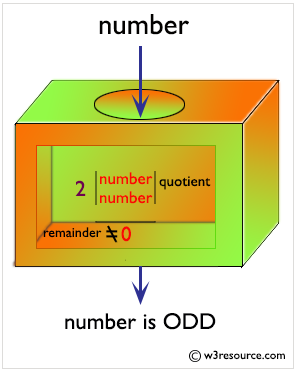
Sample Solution:
Python Code:
# Prompt the user to enter a number and convert the input to an integer
num = int(input("Enter a number: "))
# Calculate the remainder when the number is divided by 2
mod = num % 2
# Check if the remainder is greater than 0, indicating an odd number
if mod > 0:
# Print a message indicating that the number is odd
print("This is an odd number.")
else:
# Print a message indicating that the number is even
print("This is an even number.")
Sample Output:
Enter a number: 5 This is an odd number.
Explanation:
The said code prompts the user to input a number, then converts the input to an integer and assigns it to the variable 'num'. Then it calculates the remainder of 'num' and 2 and assigns it to the variable 'mod'. Next, it checks the value of 'mod'. If the value of 'mod' is greater than 0, it prints "This is an odd number." otherwise prints "This is an even number.".
Even Numbers between 1 to 100:
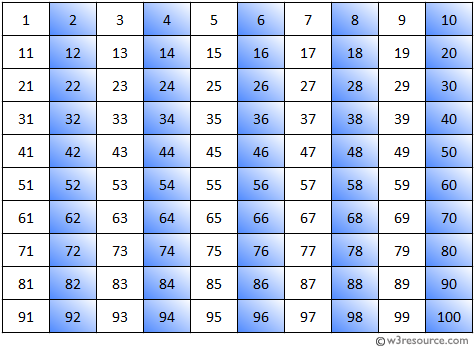
Odd Numbers between 1 to 100:
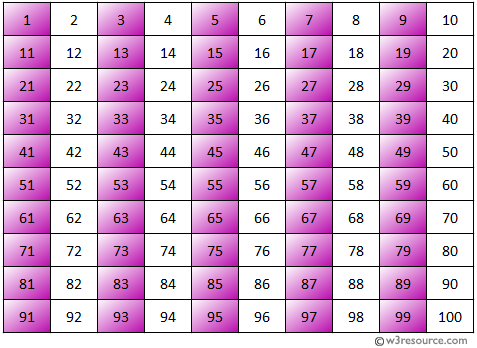
Flowchart:
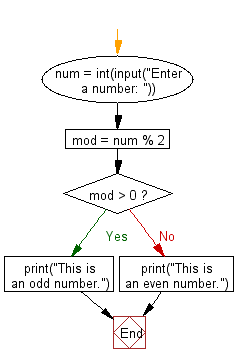
For more Practice: Solve these Related Problems:
- Write a Python program that checks whether a number is even or odd without using the modulus operator (%).
- Write a Python program to determine if a given number is odd and a multiple of 7.
- Write a script that categorizes a number as "Even and Positive," "Odd and Positive," "Even and Negative," or "Odd and Negative."
- Write a program that accepts a number and prints all even or odd numbers up to that number.
Go to:
Previous: Write a Python program to get a string which is n (non-negative integer) copies of a given string.
Next: Write a Python program to count the number 4 in a given list.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.