Python: Calculate area of a circle
Python Basic: Exercise-4 with Solution
Write a Python program that calculates the area of a circle based on the radius entered by the user.
Python: Area of a Circle
In geometry, the area enclosed by a circle of radius r is πr2. Here the Greek letter π represents a constant, approximately equal to 3.14159, which is equal to the ratio of the circumference of any circle to its diameter.
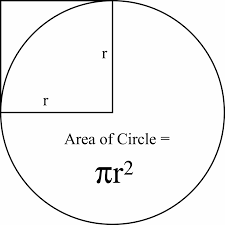
Sample Solution:
Python Code:
# Import the 'pi' constant from the 'math' module to calculate the area of a circle
from math import pi
# Prompt the user to input the radius of the circle
r = float(input("Input the radius of the circle : "))
# Calculate the area of the circle using the formula: area = π * r^2
area = pi * r ** 2
# Display the result, including the radius and calculated area
print("The area of the circle with radius " + str(r) + " is: " + str(area))
Sample Output:
Input the radius of the circle : 1.1 The area of the circle with radius 1.1 is: 3.8013271108436504
Explanation:
The said code calculates the area of a circle based on the radius entered by the user. The code uses the "math" module's pi constant and the "input" function to get the radius from the user, then it uses the formula to calculate the area of the circle.
- The first line from math import pi imports the pi constant from the math module, which is the mathematical constant. It represents the ratio of a circle's circumference to its diameter (approximately equal to 3.14).
- The second line r = float(input ("Input the radius of the circle : ")) gets the radius of the circle from the user using the input() function. input() function assigns it to the variable r, it's then cast to float, so the user can input decimal number also.
- The third line print ("The area of the circle with radius " + str(r) + " is: " + str(pi * r**2)) uses the formula to calculate the area of the circle (pi*r**2) . Then it concatenates the string and the value of the radius and area using the + operator and prints the final string.
This code is useful to calculate the area of a circle when the radius is known, it can be used in physics and mathematical calculations or in applications that require the area of a circle to be calculated.
Flowchart:
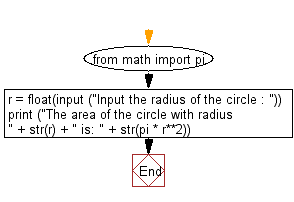
Python Code Editor:
Previous: Write a Python program to display the current date and time.
Next: Write a Python program which accepts the user's first and last name and print them in reverse order with a space between them.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics