Python: Determine profiling of Python programs
Program Profiler
Write a Python program to determine the profiling of Python programs.
Note: A profile is a set of statistics that describes how often and for how long various parts of the program executed. These statistics can be formatted into reports via the pstats module.
Sample Solution:
Python Code:
# Import the cProfile module, which provides a way to profile Python code.
import cProfile
# Define a function named 'sum'.
def sum():
# Print the result of adding 1 and 2 to the console.
print(1 + 2)
# Use cProfile to profile the 'sum' function.
cProfile.run('sum()')
Sample Output:
3 5 function calls in 0.000 seconds Ordered by: standard name ncalls tottime percall cumtime percall filename:lineno(function) 1 0.000 0.000 0.000 0.000 7aa14930-2430-11e7-807b-bd9de91b1602.py:2(sum) 1 0.000 0.000 0.000 0.000 <string>:1(<module>) 1 0.000 0.000 0.000 0.000 {built-in method builtins.exec} 1 0.000 0.000 0.000 0.000 {built-in method builtins.print} 1 0.000 0.000 0.000 0.000 {method 'disable' of '_lsprof.Profiler' objects}
Explanation:
cProfile provide deterministic profiling of Python programs. A profile is a set of statistics that describes how often and for how long various parts of the program executed.
profile.run(command, filename=None, sort=- 1): This function takes a single argument that can be passed to the exec() function, and an optional file name.
The above Python code imports the cProfile module. The sum() function defined in the code adds the numbers 1 and 2 and prints the result to the console using the print() function.
The cProfile.run() function is used to profile the execution of the sum() function. The run() function takes a string as its parameter that represents the code to be executed and profiled. In this case, it is passed the function call sum() as a string.
Flowchart:
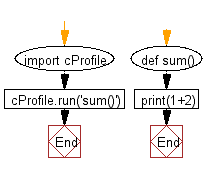
For more Practice: Solve these Related Problems:
- Write a Python program to profile a function that computes the Fibonacci sequence using recursion.
- Write a Python program to compare the execution time of two different sorting algorithms using profiling.
- Write a Python program to measure the execution time of a large matrix multiplication operation.
- Write a Python program to identify the slowest function in a given script using profiling.
Go to:
Previous: Write a Python program to print without newline or space?
Next: Write a Python program to print to stderr.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.