Python: Print to stderr
Print to STDERR
Write a Python program to print to STDERR.
Sample Solution:
Python Code:
# Import the 'print_function' feature from the '__future__' module to enable Python 3-style print function.
from __future__ import print_function
# Import the 'sys' module, which provides access to some variables used or maintained by the interpreter.
import sys
# Define a custom 'eprint' function that prints to the standard error stream.
def eprint(*args, **kwargs):
# Call the 'print' function with the provided arguments and keyword arguments.
# Use 'file=sys.stderr' to print to the standard error stream (stderr).
print(*args, file=sys.stderr, **kwargs)
# Call the 'eprint' function with the specified arguments and separator.
eprint("abc", "efg", "xyz", sep="--")
Sample Output:
abc--efg--xyz
Explanation:
__future__ is a real module, and serves three purposes:
- To avoid confusing existing tools that analyze import statements and expect to find the modules they’re importing.
- To ensure that future statements run under releases prior to 2.1 at least yield runtime exceptions (the import of __future__ will fail, because there was no module of that name prior to 2.1).
- To document when incompatible changes were introduced, and when they will be — or were — made mandatory. This is a form of executable documentation, and can be inspected programmatically via importing __future__ and examining its contents.
Sys module - This module provides access to some variables used or maintained by the interpreter and to functions that interact strongly with the interpreter. It is always available.
In the above Python exercise the eprint() function takes any number of arguments and prints them to the standard error stream (stderr) using the print() function. The file parameter is set to sys.stderr, which redirects the output to the standard error stream instead of the standard output stream.
Finally, the eprint() function is called with the arguments "abc", "efg", and "xyz", separated by the string "--". This causes the string "abc--efg--xyz" to be printed to the standard error stream.
Flowchart:
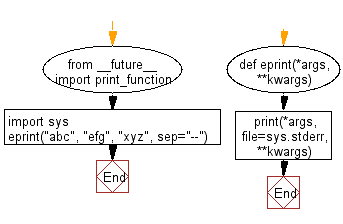
For more Practice: Solve these Related Problems:
- Write a Python program to print both normal output (STDOUT) and error messages (STDERR) simultaneously.
- Write a Python program to redirect STDERR to a log file instead of displaying it on the console.
- Write a Python program that raises an exception and prints the error message to STDERR.
- Write a Python program to suppress STDERR messages while executing a command.
Go to:
Previous: Write a Python program to determine profiling of Python programs.
Next: Write a python program to access environment variables.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.