Python Exercise: Print the 4 digit binary numbers that are divisible by 5
Python Conditional: Exercise-13 with Solution
Write a Python program that accepts a sequence of comma separated 4 digit binary numbers as its input. The program will print the numbers that are divisible by 5 in a comma separated sequence.
Pictorial Presentation:
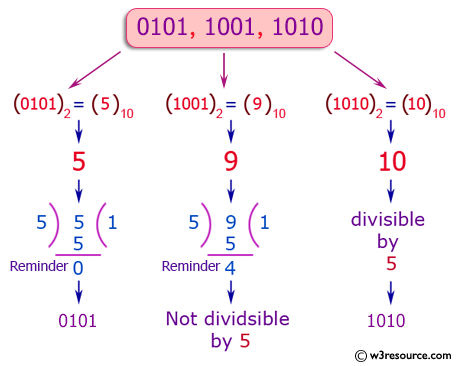
Sample Solution:
Python Code:
# Create an empty list named 'items'
items = []
# Take user input and split it into a list of strings using ',' as the delimiter
num = [x for x in input().split(',')]
# Iterate through each element 'p' in the 'num' list
for p in num:
# Convert the binary string 'p' to its decimal equivalent 'x'
x = int(p, 2)
# Check if 'x' is divisible by 5 (i.e., when divided by 5 there's no remainder)
if not x % 5:
# If 'x' is divisible by 5, add the binary string 'p' to the 'items' list
items.append(p)
# Join the elements in the 'items' list separated by ',' and print the result
print(','.join(items))
Sample Output:
0001,0010,0011,0100,0101,0110,0111 0101
Flowchart:
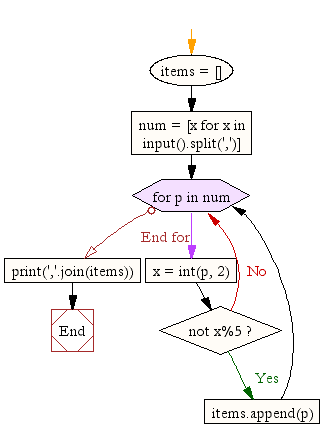
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program that accepts a sequence of lines (blank line to terminate) as input and prints the lines as output (all characters in lower case).
Next: Write a Python program that accepts a string and calculate the number of digits and letters.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/python-conditional-exercise-13.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics