Python Exercise: Calculate a dog's age in dog's years
Python Conditional: Exercise - 31 with Solution
Write a Python program to calculate a dog's age in dog years.
Note: For the first two years, a dog year is equal to 10.5 human years. After that, each dog year equals 4 human years.
Pictorial Presentation:
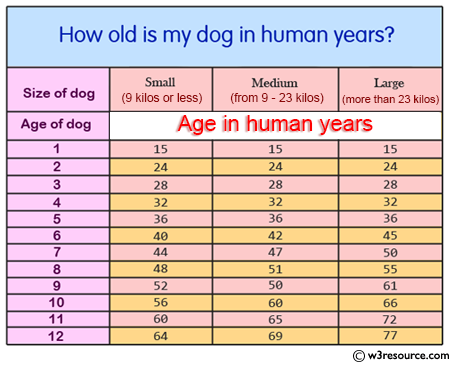
Sample Solution:
Python Code:
# Request input from the user to provide a dog's age in human years and convert it to an integer
h_age = int(input("Input a dog's age in human years: "))
# Check if the entered age is less than zero; if true, display an error message and exit the program
if h_age < 0:
print("Age must be a positive number.")
exit()
# If the entered age is 2 years or less, calculate the dog's age in dog's years using the formula 10.5 times the human years
elif h_age <= 2:
d_age = h_age * 10.5
# For ages greater than 2, calculate the dog's age in dog's years using the formula 21 plus 4 times the remaining human years after 2
else:
d_age = 21 + (h_age - 2) * 4
# Display the calculated dog's age in dog's years
print("The dog's age in dog's years is", d_age)
Sample Output:
Input a dog's age in human years: 12 The dog's age in dog's years is 61
Flowchart :
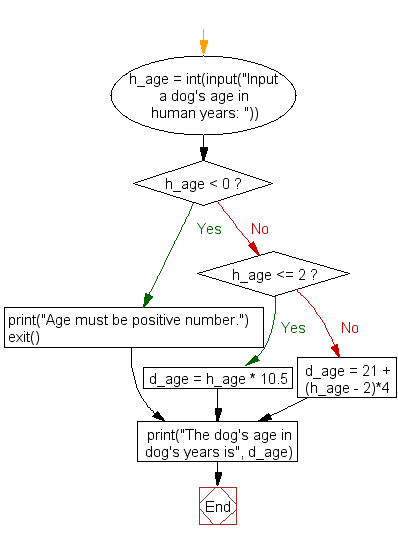
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to print alphabet pattern 'Z'.
Next: Write a Python program to check whether an alphabet is a vowel or consonant.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics