Python Exercise: Prints each item and its corresponding type from a list
Python Conditional: Exercise-7 with Solution
Write a Python program that prints each item and its corresponding type from the following list.
Sample List : datalist = [1452, 11.23, 1+2j, True, 'w3resource', (0, -1), [5, 12], {"class":'V', "section":'A'}]
Sample Solution:
Python Code:
# Create a list named 'datalist' containing various data types (int, float, complex, bool, string, tuple, list, dictionary)
datalist = [1452, 11.23, 1+2j, True, 'w3resource', (0, -1), [5, 12], {"class": 'V', "section": 'A'}]
# Iterate through each item in the 'datalist'
for item in datalist:
# Print the type of each item in the list along with the item itself
print("Type of", item, "is", type(item))
Sample Output:
Type of 1452 is <class 'int'> Type of 11.23 is <class 'float'> Type of (1+2j) is <class 'complex'> Type of True is <class 'bool'> Type of w3resource is <class 'str'> Type of (0, -1) is <class 'tuple'> Type of [5, 12] is <class 'list'> Type of {'class': 'V', 'section': 'A'} is < class 'dict'>
Flowchart:
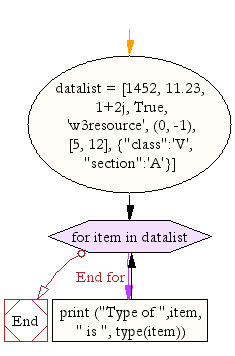
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to count the number of even and odd numbers from a series of numbers.
Next: Write a Python program that prints all the numbers from 0 to 6 except 3 and 6.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/python-conditional-exercise-7.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics