Python Exercise: Checks whether a passed string is palindrome or not
12. Check if a String is a Palindrome
Write a Python function that checks whether a passed string is a palindrome or not.
Note: A palindrome is a word, phrase, or sequence that reads the same backward as forward, e.g., madam or nurses run.
Sample Solution:
Python Code:
# Define a function named 'isPalindrome' that checks if a string is a palindrome
def isPalindrome(string):
# Initialize left and right pointers to check characters from the start and end of the string
left_pos = 0
right_pos = len(string) - 1
# Loop until the pointers meet or cross each other
while right_pos >= left_pos:
# Check if the characters at the left and right positions are not equal
if not string[left_pos] == string[right_pos]:
# If characters don't match, return False (not a palindrome)
return False
# Move the left pointer to the right and the right pointer to the left to continue checking
left_pos += 1
right_pos -= 1
# If the loop finishes without returning False, the string is a palindrome, so return True
return True
# Print the result of checking if the string 'aza' is a palindrome by calling the 'isPalindrome' function
print(isPalindrome('aza'))
Sample Output:
True
Explanation:
In the exercise above the code defines a function named "isPalindrome()" that checks whether a given string is a palindrome (reads the same forwards and backwards). It uses two pointers (left_pos and right_pos) to traverse the string from both ends towards the middle, checking each character pair. Finally, it prints the result of checking whether the string 'aza' is a palindrome (which is True in this case).
Pictorial presentation:
Flowchart:
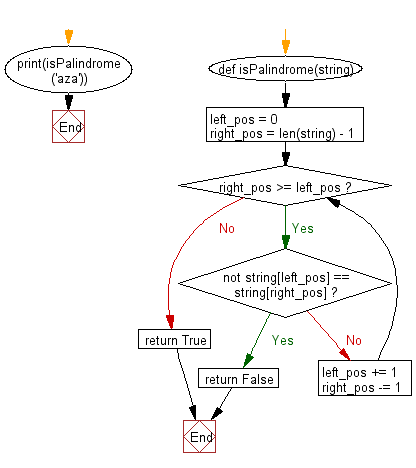
For more Practice: Solve these Related Problems:
- Write a Python function that checks if a string reads the same forwards and backwards using slicing.
- Write a Python function that iterates over a string from both ends simultaneously to determine if it is a palindrome.
- Write a Python function that ignores spaces and punctuation while checking if a sentence is a palindrome.
- Write a Python function that uses recursion to verify if a string is a palindrome.
Go to:
Previous: Write a Python function to check whether a number is perfect or not.
Next: Write a Python function that that prints out the first n rows of Pascal's triangle.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.