Python: Find the substrings within a string
21. Find Substrings
Write a Python program to find the substrings within a string.
Sample text:
'Python exercises, PHP exercises, C# exercises'
Pattern:
'exercises'
Note: There are two instances of exercises in the input string.
Sample Solution:
Python Code:
import re
text = 'Python exercises, PHP exercises, C# exercises'
pattern = 'exercises'
for match in re.findall(pattern, text):
print('Found "%s"' % match)
Sample Output:
Found "exercises" Found "exercises" Found "exercises"
Pictorial Presentation:
Flowchart:
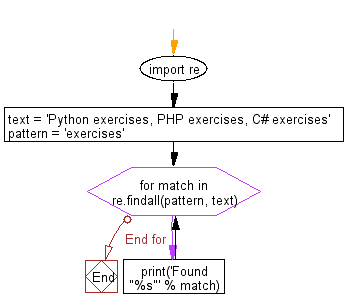
For more Practice: Solve these Related Problems:
- Write a Python program to extract all occurrences of a specific substring pattern from a text and print them.
- Write a Python script to search a string for any substrings that match a given pattern and then list them.
- Write a Python program to identify and print all substrings within a string that are enclosed by specific delimiters.
- Write a Python program to scan a text for substrings that meet certain criteria and then return a list of those substrings.
Go to:
Previous: Write a Python program to search a literals string in a string and also find the location within the original string where the pattern occurs.
Next: Write a Python program to find the occurrence and position of the substrings within a string.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.