Python: Remove words from a string of length between 1 and a given number
49. Remove Short Words
Write a Python program to remove words from a string of length between 1 and a given number.
Sample Solution:
Python Code:
import re
text = "The quick brown fox jumps over the lazy dog."
# remove words between 1 and 3
shortword = re.compile(r'\W*\b\w{1,3}\b')
print(shortword.sub('', text))
Sample Output:
quick brown jumps over lazy.
Pictorial Presentation:
Flowchart:
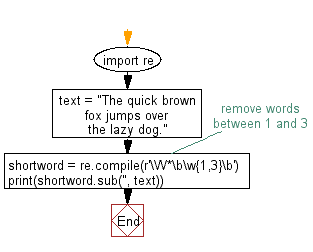
For more Practice: Solve these Related Problems:
- Write a Python program to remove all words from a string that have a length between 1 and a given threshold.
- Write a Python script to filter out words shorter than a specified number of characters from a sentence.
- Write a Python program to delete words of length less than 3 from a given text and print the cleaned string.
- Write a Python program to remove all words from a text that are below a certain length and then output the modified string.
Go to:
Previous: Write a Python program to check a decimal with a precision of 2.
Next: Write a Python program to remove the parenthesis area in a string.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.