Python: Return unique strings from a list of strings
Python sets: Exercise-30 with Solution
Write a Python program to remove all duplicates from a given list of strings and return a list of unique strings. Use the Python set data type.
Sample Solution:
Python Code:
# Define a function 'remove_duplicates' that takes a list of 'strings' as input.
def remove_duplicates(strings):
# Convert the 'strings' list into a set to remove duplicate elements.
return list(set(strings))
# Define a list of strings 'strs' for testing.
strs = ['foo', 'bar', 'abc', 'foo', 'qux', 'bar', 'baz']
print("Original list of strings:")
print(strs)
# Call the 'remove_duplicates' function and print the list of unique strings.
print("List of strings after removing duplicates:")
print(remove_duplicates(strs))
# Repeat the process for a different input list.
strs = ["Python", "Exercises", "Practice", "Solution", "Exercises"]
print("\nOriginal list of strings:")
print(strs)
# Call the 'remove_duplicates' function and print the list of unique strings.
print("List of strings after removing duplicates:")
print(remove_duplicates(strs))
Sample Output:
Original list of strings: ['foo', 'bar', 'abc', 'foo', 'qux', 'bar', 'baz'] strs of strings after removing duplicates: ['abc', 'qux', 'foo', 'baz', 'bar'] Original list of strings: ['Python', 'Exercises', 'Practice', 'Solution', 'Exercises'] List of strings after removing duplicates: ['Solution', 'Python', 'Exercises', 'Practice']
Flowchart:
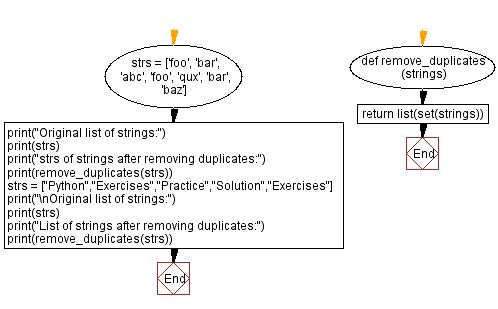
Python Code Editor:
Previous: Third largest number from a list of numbers using set.
Next: Python Collections Exercise Home.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics