Python Exercises: Remove punctuations from a string
Remove punctuation from string.
From Wikipedia,
Punctuation is the use of spacing, conventional signs, and certain typographical devices as aids to the understanding and correct reading of written text. The marks, such as full stop, comma, and brackets, used in writing to separate sentences and their elements and to clarify meaning.
Write a Python program to remove punctuation from a given string.
Sample Solution-1:
Python Code:
# Import the 'string' module for accessing a string of punctuation characters
import string
# Define a function to remove punctuation characters from a given string
def remove_punctuations(text):
# Iterate through each punctuation character and replace it with an empty string
for c in string.punctuation:
text = text.replace(c, "")
# Return the modified string without punctuation
return text
# Initialize a string with punctuation
text = "String! With. Punctuation?"
# Print the original text
print("Original text:")
print(text)
# Call the function to remove punctuation characters and print the result
result = remove_punctuations(text)
print("After removing Punctuations from the said string:")
print(result)
Sample Output:
Original text: String! With. Punctuation? After removing Punctuations from the said string: String With Punctuation
Flowchart:
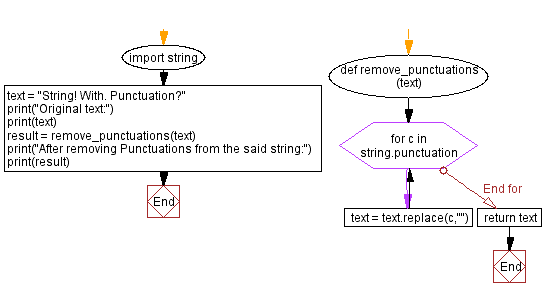
Sample Solution-2:
Python Code:
# Import the 'string' module for accessing a string of punctuation characters
import string
# Define a function to remove punctuation characters from a given string using translation
def remove_punctuations(text):
# Use the translate method with str.maketrans to remove punctuation characters
return text.translate(str.maketrans('', '', string.punctuation))
# Initialize a string with punctuation
text = "String! With. Punctuation?"
# Print the original text
print("Original text:")
print(text)
# Call the function to remove punctuation characters and print the result
result = remove_punctuations(text)
print("After removing Punctuations from the said string:")
print(result)
Sample Output:
Original text: String! With. Punctuation? After removing Punctuations from the said string: String With Punctuation
Flowchart:
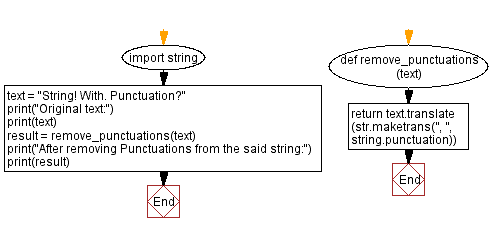
Sample Solution-3:
Python Code:
# Define a function to remove specific punctuation characters from a given string
def remove_punctuations(text):
# Define a string containing specific punctuation characters to be removed
punc_list = '''!()-[]{};:'"\,<>./?@#$%^&*_~'''
# Initialize an empty string to store the result after removing punctuation characters
result = ""
# Iterate through each character in the input string
for char in text:
# Check if the character is not in the specified punctuation list
if char not in punc_list:
# If true, add the character to the result string
result = result + char
# Return the modified string without specified punctuation
return result
# Initialize a string with various punctuation characters
text = "@^&$String! With.-- Punctuation?"
# Print the original text
print("Original text:")
print(text)
# Call the function to remove specified punctuation characters and print the result
result = remove_punctuations(text)
print("\nAfter removing Punctuations from the said string:")
print(result)
Sample Output:
Original text: @^&$String! With.-- Punctuation? After removing Punctuations from the said string: String With Punctuation
Flowchart:
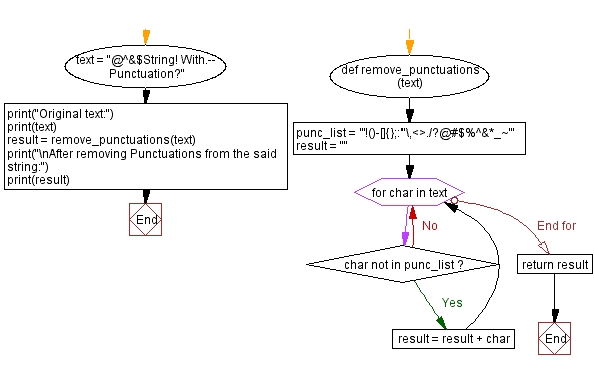
For more Practice: Solve these Related Problems:
- Write a Python program to remove all punctuation characters from a string using the string.punctuation constant.
- Write a Python program to use regular expressions to filter out punctuation from the given text.
- Write a Python program to iterate through a string and construct a new string that excludes any punctuation.
- Write a Python program to implement a function that cleans a string by replacing punctuation with spaces and then stripping extra whitespace.
Go to:
Previous Python Exercise: Add two strings as they are numbers (Positive integer values).
Next Python Exercise: Replace a word with hash characters in a string.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.