Python Exercises: Capitalize the first letter and lowercases the rest
Python String: Exercise-104 with Solution
Capitalize first letter of words.
Write a Python program that capitalizes the first letter and lowercases the remaining letters in a given string.
Sample Data:
(“Red Green WHITE) -> “Red Green White”
(“w3resource”) -> “W3resource”
(“dow jones industrial average”) -> “Dow Jones Industrial Average”
Sample Solution-1:
Python Code:
# Define a function to capitalize the first letter and lowercase the rest of each word in a string
def test(strs):
# Use a generator expression to capitalize each word and join them with spaces
return ' '.join(word.capitalize() for word in strs.split())
# Initialize a string with words in various cases
text = "Red Green WHITE"
print("Original string:", text)
# Print a message indicating the capitalization of the first letter in each word
print("Capitalize the first letter and lowercases the rest:")
# Call the function to capitalize words and print the result
print(test(text))
# Repeat the process with a different string
text = "w3resource"
print("\nOriginal string:", text)
# Print a message indicating the capitalization of the first letter in each word
print("Capitalize the first letter and lowercases the rest:")
# Call the function to capitalize words and print the result
print(test(text))
# Repeat the process with another different string
text = "dow jones industrial average"
print("\nOriginal string:", text)
# Print a message indicating the capitalization of the first letter in each word
print("Capitalize the first letter and lowercases the rest:")
# Call the function to capitalize words and print the result
print(test(text))
Sample Output:
Original string: Red Green WHITE Capitalize the first letter and lowercases the rest: Red Green White Original string: w3resource Capitalize the first letter and lowercases the rest: W3resource Original string: dow jones industrial average Capitalize the first letter and lowercases the rest: Dow Jones Industrial Average
Flowchart:
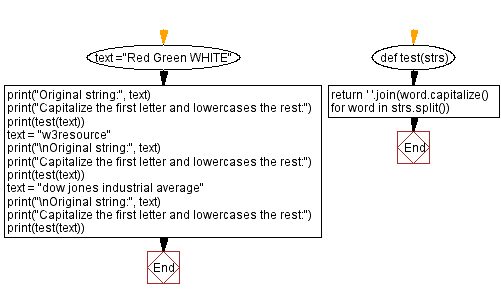
Sample Solution-2:
Python Code:
# Define a function to capitalize the first letter and lowercase the rest of each word in a string
def test(strs):
# Use a generator expression to capitalize the first letter and lowercase the rest of each word
return ' '.join(i[0].upper() + i[1:].lower() for i in strs.split())
# Initialize a string with words in various cases
text = "Red Green WHITE"
print("Original string:", text)
# Print a message indicating the capitalization of the first letter in each word
print("Capitalize the first letter and lowercases the rest:")
# Call the function to capitalize words and print the result
print(test(text))
# Repeat the process with a different string
text = "w3resource"
print("\nOriginal string:", text)
# Print a message indicating the capitalization of the first letter in each word
print("Capitalize the first letter and lowercases the rest:")
# Call the function to capitalize words and print the result
print(test(text))
# Repeat the process with another different string
text = "dow jones industrial average"
print("\nOriginal string:", text)
# Print a message indicating the capitalization of the first letter in each word
print("Capitalize the first letter and lowercases the rest:")
# Call the function to capitalize words and print the result
print(test(text))
Sample Output:
Original string: Red Green WHITE Capitalize the first letter and lowercases the rest: Red Green White Original string: w3resource Capitalize the first letter and lowercases the rest: W3resource Original string: dow jones industrial average Capitalize the first letter and lowercases the rest: Dow Jones Industrial Average
Flowchart:
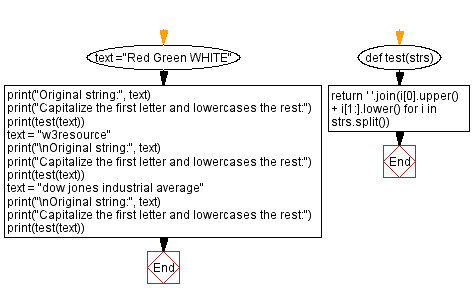
Python Code Editor:
Previous Python Exercise: Replace a word with hash characters in a string.
Next Python Exercise: Extract the name from an Email address.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/string/python-data-type-string-exercise-104.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics