Python Exercises: Extract the name from an Email address
Extract name from email address.
From Wikipedia –
An email address consists of two parts, a local part[a] and a domain; if the domain is a domain name rather than an IP address then the SMTP client uses the domain name to look up the mail exchange IP address. The general format of an email address is local-part@domain, e.g. jsmith@[192.168.1.2], [email protected].
Write a Python program to extract and display the name from a given Email address.
Sample Data:
("[email protected]") -> ("john")
("[email protected]") -> ("johnsmith")
("[email protected]") -> ("fullyqualifieddomain")
Sample Solution-1:
Python Code:
# Define a function to extract the name from an email address
def test(email_address):
# Find the index of the '@' symbol in the email address
r = email_address.index("@")
# Use a generator expression to join alphabetic characters before the '@' symbol
return "".join(l for l in email_address[:r] if l.isalpha())
# Initialize an email address
email_address = "[email protected]"
print("Original Email:", email_address)
# Print a message indicating the extraction of the name from the email address
print("Extract the name from the said Email address:")
# Call the function to extract the name and print the result
print(test(email_address))
# Repeat the process with a different email address
email_address = "[email protected]"
print("\nOriginal Email:", email_address)
# Print a message indicating the extraction of the name from the email address
print("Extract the name from the said Email address:")
# Call the function to extract the name and print the result
print(test(email_address))
# Repeat the process with another different email address
email_address = "[email protected]@example.com"
print("\nOriginal Email:", email_address)
# Print a message indicating the extraction of the name from the email address
print("Extract the name from the said Email address:")
# Call the function to extract the name and print the result
print(test(email_address))
# Repeat the process with yet another different email address
email_address = "[email protected]"
print("\nOriginal Email:", email_address)
# Print a message indicating the extraction of the name from the email address
print("Extract the name from the said Email address:")
# Call the function to extract the name and print the result
print(test(email_address))
Sample Output:
Original Email: [email protected] Extract the name from the said Email address: john Original Email: [email protected] Extract the name from the said Email address: johnsmith Original Email: [email protected]@example.com Extract the name from the said Email address: disposablestyleemailwithsymbol Original Email: [email protected] Extract the name from the said Email address: fullyqualifieddomain
Flowchart:
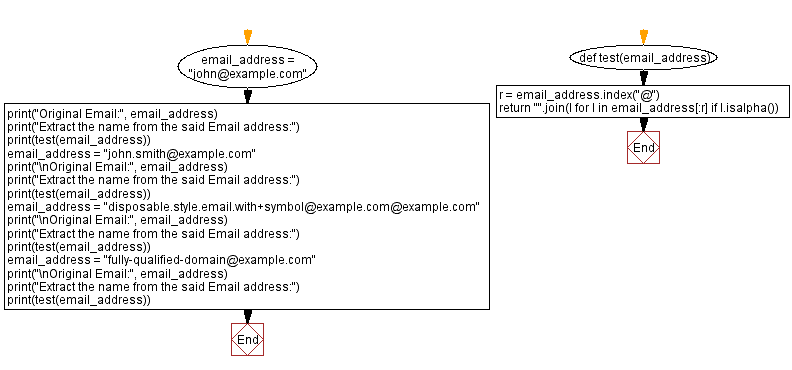
Sample Solution-2:
Python Code:
# Define a function to extract the name from an email address
def test(email_address):
# Split the email address using '@' and take the first part
data = email_address.split("@")[0]
# Initialize an empty string to store alphabetic characters
result = ""
# Iterate through each character in the data
for i in data:
# Check if the character is alphabetic
if i.isalpha():
# If true, add the character to the result string
result += i
# Return the result containing only alphabetic characters
return result
# Initialize an email address
email_address = "[email protected]"
print("Original Email:", email_address)
# Print a message indicating the extraction of the name from the email address
print("Extract the name from the said Email address:")
# Call the function to extract the name and print the result
print(test(email_address))
# Repeat the process with a different email address
email_address = "[email protected]"
print("\nOriginal Email:", email_address)
# Print a message indicating the extraction of the name from the email address
print("Extract the name from the said Email address:")
# Call the function to extract the name and print the result
print(test(email_address))
# Repeat the process with another different email address
email_address = "[email protected]@example.com"
print("\nOriginal Email:", email_address)
# Print a message indicating the extraction of the name from the email address
print("Extract the name from the said Email address:")
# Call the function to extract the name and print the result
print(test(email_address))
# Repeat the process with yet another different email address
email_address = "[email protected]"
print("\nOriginal Email:", email_address)
# Print a message indicating the extraction of the name from the email address
print("Extract the name from the said Email address:")
# Call the function to extract the name and print the result
print(test(email_address))
Sample Output:
Original Email: [email protected] Extract the name from the said Email address: john Original Email: [email protected] Extract the name from the said Email address: johnsmith Original Email: [email protected]@example.com Extract the name from the said Email address: disposablestyleemailwithsymbol Original Email: [email protected] Extract the name from the said Email address: fullyqualifieddomain
Flowchart:
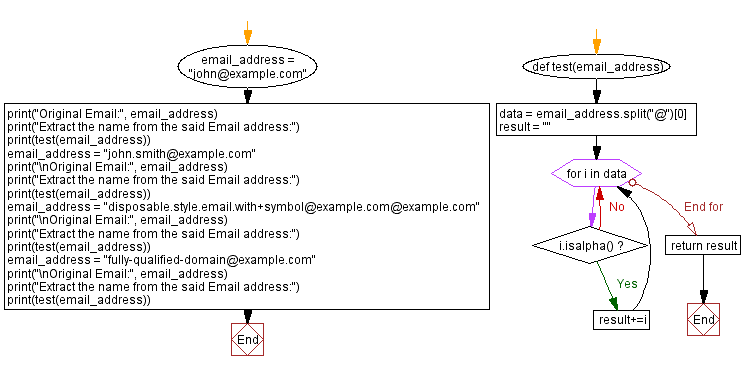
For more Practice: Solve these Related Problems:
- Write a Python program to extract the username from an email address by splitting at the '@' symbol.
- Write a Python program to remove dots and other non-alphanumeric characters from the username portion of an email address.
- Write a Python program to use slicing and string methods to extract the part of an email before the '@' symbol and remove any punctuation.
- Write a Python program to implement a function that returns the cleaned-up username from a provided email address.
Go to:
Previous Python Exercise: Capitalize the first letter and lowercases the rest.
Next Python Exercise: Replace repeated characters with single letters.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.