Python: Split a multiline string into a list of lines
Python String: Exercise-99 with Solution
Split multiline string to lines.
Write a Python program to split a multi-line string into a list of lines.
- Use str.split() and '\n' to match line breaks and create a list.
- str.splitlines() provides similar functionality to this snippet.
Sample Solution:
Python Code:
# Define a function to split a string into a list of lines based on newline characters
def split_lines(s):
# Use the split() method with '\n' as the delimiter to create a list of lines
return s.split('\n')
# Print a message indicating the original string
print("Original string:")
# Print a multiline string for demonstration purposes
print("This\nis a\nmultiline\nstring.\n")
# Print a message indicating the splitting of the multiline string into a list of lines
print("Split the said multiline string into a list of lines:")
# Call the function to split the multiline string and print the result
print(split_lines('This\nis a\nmultiline\nstring.\n'))
Sample Output:
Original string: This is a multiline string. Split the said multiline string into a list of lines: ['This', 'is a', 'multiline', 'string.', '']
Flowchart:
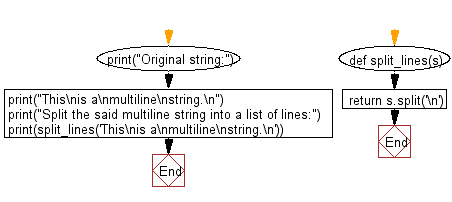
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to decapitalize the first letter of a given string.
Next: Write a Python program to check whether any word in a given sting contains duplicate characrters or not. Return True or False.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/string/python-data-type-string-exercise-99.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics