Python Multi-threading and Concurrency: Multi-threaded factorial calculation
Python Multi-threading: Exercise-4 with Solution
Write a Python program to calculate the factorial of a number using multiple threads.
Sample Solution:
Python Code:
import threading
def factorial_of_a_anumber(n):
result = 1
for i in range(1, n+1):
result *= i
return result
def calculate_factorial(n):
print(f"\nCalculating factorial of {n} in thread {threading.current_thread().name}")
result = factorial_of_a_anumber(n)
print(f"Factorial of {n} is {result} in thread {threading.current_thread().name}")
# Factorial of 12
n = 12
# Create threads for factorial calculation
thread1 = threading.Thread(target=calculate_factorial, args=(n,))
thread2 = threading.Thread(target=calculate_factorial, args=(n,))
# Start the threads
thread1.start()
thread2.start()
# Wait for the threads to complete
thread1.join()
thread2.join()
Sample Output:
Calculating factorial of 12 in thread Thread-6 Factorial of 12 is 479001600 in thread Thread-6 Calculating factorial of 12 in thread Thread-7 Factorial of 12 is 479001600 in thread Thread-7
Explanation:
In this program, we define a "factorial_of_a_number()" function that calculates the factorial of a number n using a sequential loop. We also define a "calculate_factorial()" function that takes a number as input, prints a message indicating the current thread. It calls the "factorial_of_a_number()" function, and prints the result.
We then create two threads, thread1 and thread2, targeting the "calculate_factorial()" function with the same number as an argument. We start the threads using the start() method, which initiates their parallel execution. Finally, we use the join() method to wait for threads to complete.
When you run this program, it calculates the factorial of the given number in two separate threads.
Flowchart:
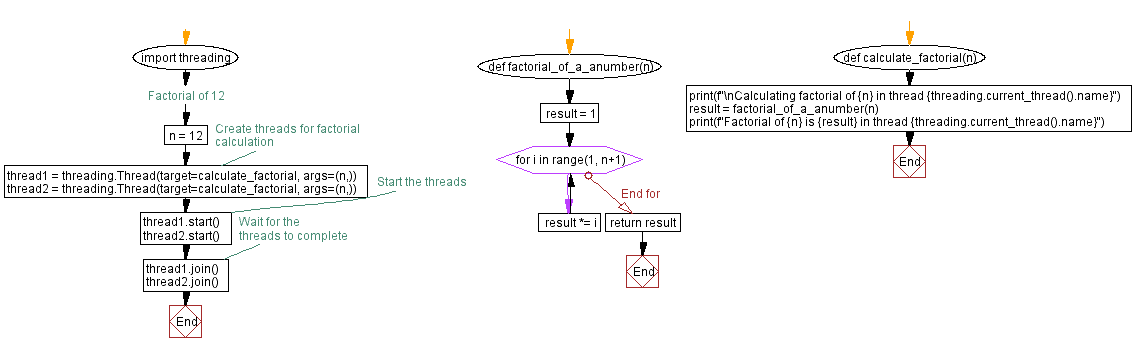
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Concurrent even and odd number printing.
Next: Multi-threaded merge sort implementation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/threading/python-multi-threading-exercise-4.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics