Creating a colorful canvas drawing program with Python and Tkinter
Write a Python program that allows the user to select a color and draw on the Canvas with that color using Tkinter.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import colorchooser
def choose_color():
color = colorchooser.askcolor()[1]
if color:
current_color.set(color)
def start_drawing(event):
global last_x, last_y, drawing
last_x, last_y = event.x, event.y
drawing = True
def draw(event):
global last_x, last_y
if drawing:
x, y = event.x, event.y
canvas.create_line(last_x, last_y, x, y, fill=current_color.get(), width=2)
last_x, last_y = x, y
def stop_drawing(event):
global drawing
drawing = False
root = tk.Tk()
root.title("Colorful Canvas")
canvas = tk.Canvas(root, bg="white")
canvas.pack(fill=tk.BOTH, expand=True)
current_color = tk.StringVar()
current_color.set("black")
color_button = tk.Button(root, text="Choose Color", command=choose_color)
color_button.pack()
drawing = False # Initialize the drawing state variable
canvas.bind("<Button-1>", start_drawing)
canvas.bind("<B1-Motion>", draw)
canvas.bind("<ButtonRelease-1>", stop_drawing)
root.mainloop()
Explanation:
In the exercise above -
- First we create a "Canvas" widget where the user can draw.
- There is a "Choose Color" button that opens a color chooser dialog when clicked. The chosen color is stored in the 'current_color' variable.
- We use the "bind()" method to capture mouse events for starting, drawing, and stopping the drawing process. When the user clicks and drags the mouse, it draws lines on the canvas using the selected color.
- The color is set dynamically as per the user's choice from the color chooser dialog.
- Now using the selected color, the user can draw on the canvas.
Output:
Flowchart:
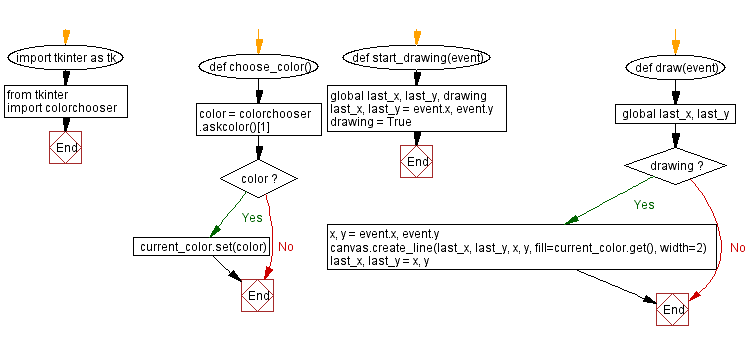
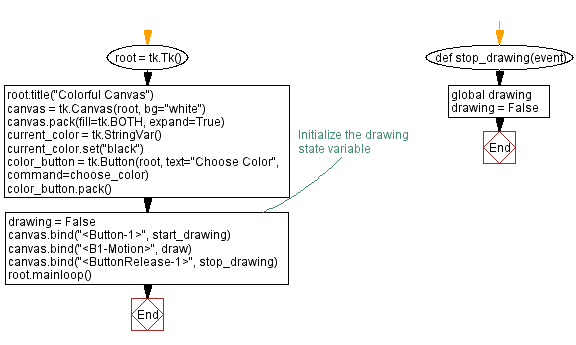
Go to:
Previous: Creating a versatile drawing program with Python and tkinter.
Next: Creating an image zooming application in Python with Tkinter.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.