Python Tkinter: Creating a simple information dialog
Write a Python program that displays a simple information dialog using Tkinter.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import messagebox
def show_info_dialog():
messagebox.showinfo("Information", "This is a simple information dialog.")
root = tk.Tk()
root.title("Information Dialog Example")
info_button = tk.Button(root, text="Show Info Dialog", command=show_info_dialog)
info_button.pack(padx=20, pady=20)
root.mainloop()
Explanation:
In the exercise above -
- import tkinter as tk - Import the required library for creating the GUI components
- from tkinter import messagebox – Import messagebox for displaying the information dialog.
- def show_info_dialog(): - Define a function "show_info_dialog()" that displays an information dialog using messagebox.showinfo(). It takes two arguments: the dialog title ("Information") and the message to display ("This is a simple information dialog.").
- Next it create the main window using tk.Tk() and set its title.
- info_button = tk.Button(root, text="Show Info Dialog", command=show_info_dialog) - Create a button named 'info_button' that, when clicked, calls the “show_info_dialog()” function to display the information dialog.
- info_button.pack(padx=20, pady=20) - Display the information dialog.
- root.mainloop() - The main event loop, root.mainloop(), starts the Tkinter application.
Sample Output:
Flowchart:
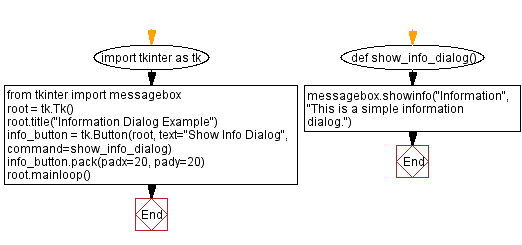
Go to:
Previous: Python Tkinter Dialogs and File Handling Home.
Next: Creating an exit confirmation dialog.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.