Python Tkinter directory file list viewer
Write a Python program that prompts the user to select a directory and then lists all the files in that directory using a Treeview widget using Tkinter.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import filedialog
import os
import tkinter.ttk as ttk
def browse_directory():
folder_path = filedialog.askdirectory()
if folder_path:
current_dir.set(folder_path)
list_files(folder_path)
def list_files(folder_path):
for item in tree_view.get_children():
tree_view.delete(item)
for filename in os.listdir(folder_path):
if os.path.isfile(os.path.join(folder_path, filename)):
tree_view.insert("", "end", values=(filename,))
root = tk.Tk()
root.title("File List Viewer")
current_dir = tk.StringVar()
browse_button = tk.Button(root, text="Browse Directory", command=browse_directory)
browse_button.pack(pady=10)
folder_label = tk.Label(root, textvariable=current_dir)
folder_label.pack()
tree_view = ttk.Treeview(root, columns=("Files",), show="headings", selectmode="browse")
tree_view.heading("Files", text="Files in Directory")
tree_view.pack(padx=20, pady=20, fill="both", expand=True)
root.mainloop()
Explanation:
In the exercise above -
- Import "tkinte"r as "tk", "filedialog", and "os" for creating the GUI components, opening the directory dialog, and listing files in the directory.
- Define the "browse_directory()" function, which opens a directory dialog using "filedialog.askdirectory()" function. This dialog allows the user to select a directory. If a directory is selected, it sets the current directory variable current_dir and calls the list_files() function to list the files in the selected directory.
- Define the "list_files()" function, which clears the existing items in the Treeview widget using "tree_view.delete()" function and then lists the files in the selected directory using os.listdir(). It inserts each file into the Treeview widget.
- Create the main Tkinter window, set its title, and create a "Browse Directory" button that calls the "browse_directory()" function when clicked.
- Display the selected folder path using a label.
- Create a Treeview widget with a single column to display a list of files.
- The main event loop, root.mainloop(), starts the Tkinter application.
Sample Output:
Flowchart:
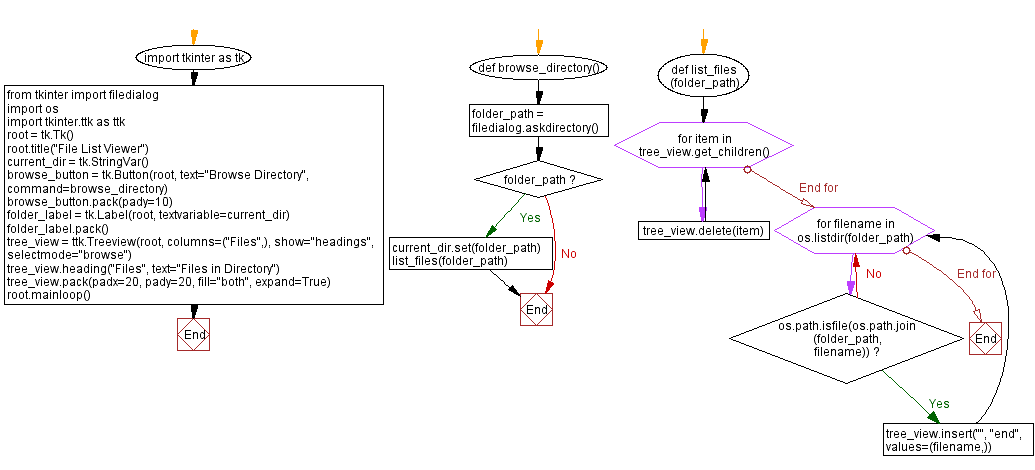
Go to:
Previous: Python Tkinter text editor with file dialogs.
Next: Python Tkinter calculator with file saving.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.