Python Tkinter timer App - Countdown timer
Python Tkinter Events and Event Handling: Exercise-10 with Solution
Write a Python program that builds a timer application using Tkinter. The application starts a countdown when a "Start" button is clicked and stops when a "Stop" button is clicked.
Sample Solution:
Python Code:
import tkinter as tk
class TimerApp:
def __init__(self, root):
self.root = root
self.root.title("Timer App")
# Initialize timer variables
self.seconds = 0
self.timer_running = False
# Create a label to display the timer
self.timer_label = tk.Label(root, text="00:00", font=("Helvetica", 48))
self.timer_label.pack(pady=20)
# Create Start and Stop buttons
self.start_button = tk.Button(root, text="Start", command=self.start_timer)
self.stop_button = tk.Button(root, text="Stop", command=self.stop_timer)
self.start_button.pack(side=tk.LEFT, padx=10)
self.stop_button.pack(side=tk.LEFT, padx=10)
# Update the timer display
self.update_timer()
def start_timer(self):
if not self.timer_running:
self.timer_running = True
self.update_timer()
def stop_timer(self):
self.timer_running = False
def update_timer(self):
if self.timer_running:
self.seconds += 1
minutes = self.seconds // 60
seconds = self.seconds % 60
time_str = f"{minutes:02}:{seconds:02}"
self.timer_label.config(text=time_str)
self.root.after(1000, self.update_timer) # Update every 1 second
if __name__ == "__main__":
root = tk.Tk()
app = TimerApp(root)
root.mainloop()
Explanation:
In the exercise above -
- Import the "tkinter" library.
- Create a "TimerApp" class that represents the timer application.
- The 'init' method initializes the main application window and timer variables. Additionally, labels are created for displaying the timer and buttons for starting and stopping it.
- The "start_timer()" method starts the timer by setting the timer_running flag to True.
- The "stop_timer()" method stops the timer by setting the timer_running flag to False.
- The "update_timer()" method is called repeatedly if the timer is running. The timer is incremented by one second and the timer display is updated.
- Finally, start the Tkinter main loop with "root.mainloop()".
Output:
Flowchart:
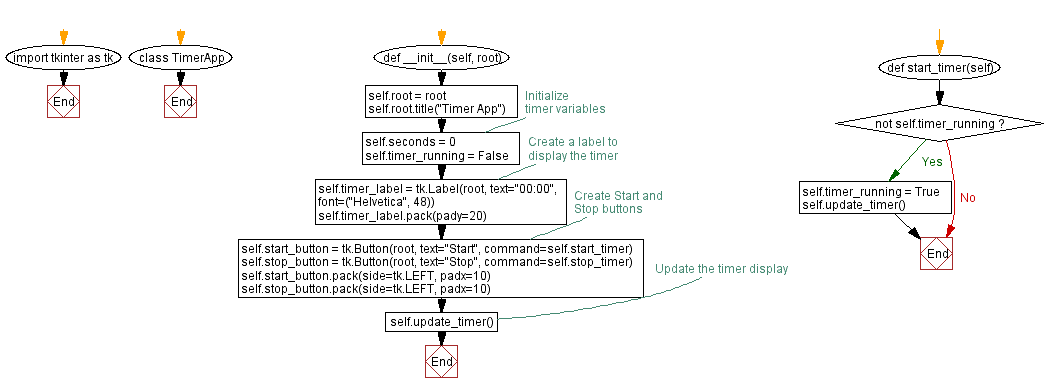
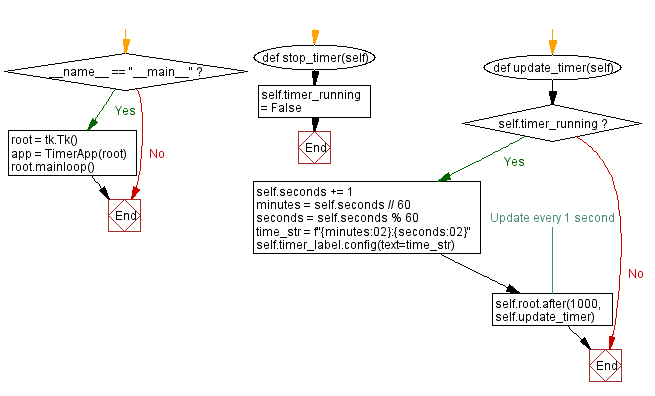
Python Code Editor:
Previous: Freehand drawing and undo.
Next: Create menu options.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics