Create a Python Tkinter auditorium reservation system
Python tkinter layout management: Exercise-11 with Solution
Write a Python program that implements a seating reservation system for an auditorium, arranging seat buttons in a grid layout.
Sample Solution:
Python Code:
import tkinter as tk
class AuditoriumReservationSystem:
def __init__(self, parent):
self.parent = parent
self.parent.title("Auditorium Reservation")
# Auditorium layout (number of rows and columns)
self.rows = 5
self.columns = 6
# Create a Frame to hold seat buttons
self.seating_frame = tk.Frame(parent)
self.seating_frame.pack()
# Create and display seat buttons in a grid
self.create_seats()
def create_seats(self):
self.seat_buttons = []
for row in range(self.rows):
seat_row = []
for col in range(self.columns):
seat_button = tk.Button(self.seating_frame, text=f"Seat {row+1}-{col+1}", width=8, height=2, command=lambda r=row, c=col: self.reserve_seat(r, c))
seat_button.grid(row=row, column=col, padx=5, pady=5)
seat_row.append(seat_button)
self.seat_buttons.append(seat_row)
def reserve_seat(self, row, col):
seat_button = self.seat_buttons[row][col]
if seat_button['state'] == 'normal':
seat_button.configure(bg='lightgreen', state='disabled')
print(f"Reserved Seat {row+1}-{col+1}")
else:
print(f"Seat {row+1}-{col+1} is already reserved.")
if __name__ == "__main__":
parent = tk.Tk()
app = AuditoriumReservationSystem(parent)
parent.mainloop()
Explanation:
In the exercise above -
- First we create a class “AuditoriumReservationApp” to manage the reservation system.
- The auditorium layout is specified with a predefined number of rows and columns.
- The "create_seats()" method creates seat buttons as Tkinter buttons and arranges them in a grid using nested loops.
- The "reserve_seat()" method handles seat reservations. When a seat button is clicked, it turns 'lightgreen' and disables further clicks.
Sample Output:
Reserved Seat 2-2 Reserved Seat 4-4 Reserved Seat 4-6 Reserved Seat 3-6 Reserved Seat 4-2![]()
Flowchart:
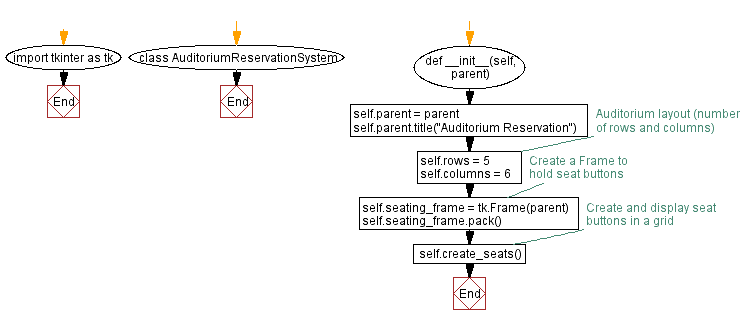
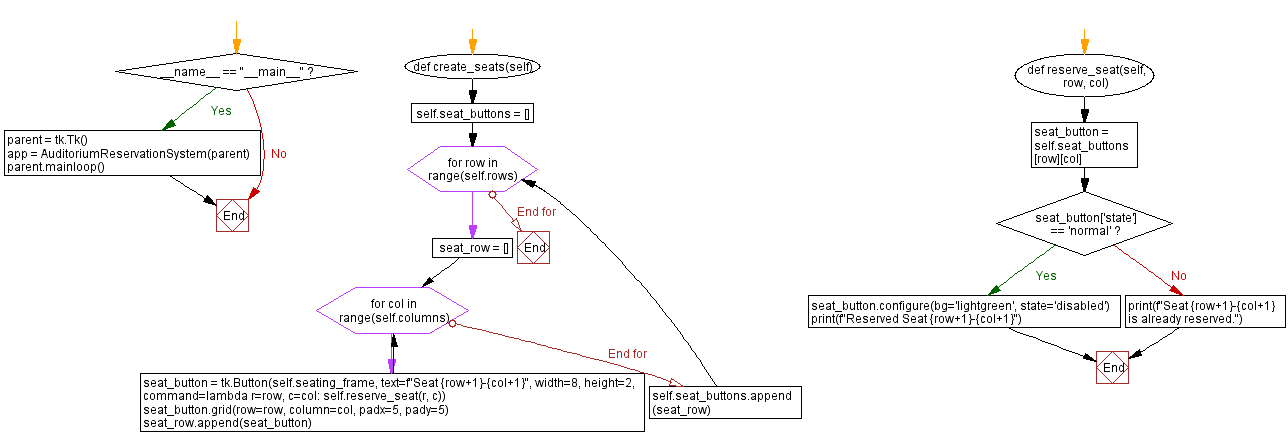
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/tkinter/python-tkinter-layout-management-exercise-11.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics