Python Exercise: Count the elements in a list until an element is a tuple
24. Count Elements in a List Until an Element is a Tuple
Write a Python program to count the elements in a list until an element is a tuple.
Sample Solution:
Python Code:
# Create a list 'num' that contains a sequence of numbers and a tuple.
num = [10, 20, 30, (10, 20), 40]
# Initialize a counter 'ctr' to keep track of the index of the first tuple in the list.
ctr = 0
# Iterate through each element 'n' in the 'num' list.
for n in num:
# Check if 'n' is an instance of a tuple.
if isinstance(n, tuple):
# If 'n' is a tuple, exit the loop.
break
# Increment the counter 'ctr' for non-tuple elements.
ctr += 1
# Print the value of the 'ctr' variable, which represents the index of the first tuple in the list.
print(ctr)
Sample Output:
3
Flowchart:
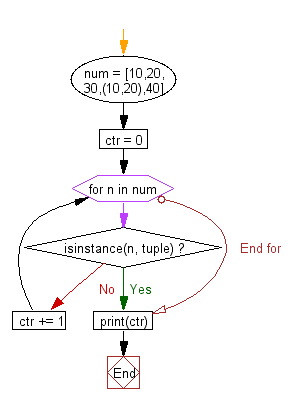
For more Practice: Solve these Related Problems:
- Write a Python program to iterate over a list and count elements until the first tuple is encountered.
- Write a Python program to use a loop to traverse a list and break the loop when an element of type tuple is found, returning the count.
- Write a Python program to implement a function that returns the index of the first tuple in a list.
- Write a Python program to use enumerate() to count elements until an element is a tuple and then output the count.
Go to:
Previous: Write a Python program to sort a tuple by its float element.
Next: Write a Python program convert a given string list to a tuple.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.